Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial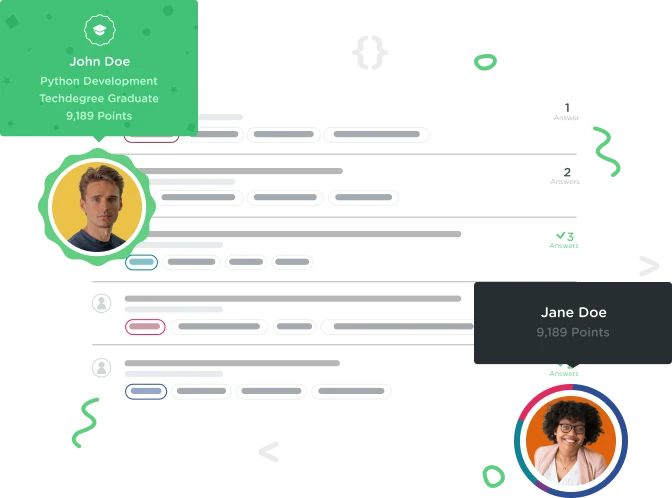

CRIS ACOSTA
2,121 PointsWhaaaat???? My overthinking did it to me again.
Wow! I spent like 4 days again on this veeeeeery simple challenge. My overthinking got the best of me again, instead of just writing an an array of objects I ended up creating a code that prints the objects into the DOM and took me 4 days to make it work - at this pace, I may not become a front-end developer before the end of the year. Anyway, since I already created the code might as well show you for your scrutiny. Feedback is always appreciated my senpais!
COOOODE
var students = [
{name : 'Cris A.', track: 'Full-stack Javascript developer', Achievements: 500, Points: 50000 },
{name: 'Dave M.', track: 'Full-stack Javascript developer', Achievements: 230, Points: 77469},
{name: 'Dylan I.', track: 'iOS DEVELOPER', Achievements: 346, Points: 35035},
{name: 'Bucky R.', track: 'PYTHON', Achievements: 325, Points: 35416},
{name: 'MPJ', track: 'PHP', Achievements: 456, Points: 42167}
];
var objContainer = {};
function print(message) {
var divOutput = document.getElementById('output');
divOutput.innerHTML = message;
}
function studentObj(obj){
objContainer = '<ul>';
for(i=0; i<obj.length; i++){
for(prop in obj[i]){
console.log(prop + ": " + obj[i][prop]);
objContainer += '<li>' + prop + ': ' + obj[i][prop] + '</li>';
}
}objContainer += '</ul>';
return objContainer;
}
print(studentObj(students));
By the way, how do we save our own codes here?
3 Answers

Matthew Lanin
Full Stack JavaScript Techdegree Student 8,003 PointsYeah, it looks like things got a little convoluted. Happens to me all the time, my new mantra when trying to form a plan of attack is KISS, or, "Keep It Simple, Stupid." It's good because it reminds you to try to find a simple solution, and you challenge your own intelligence and thus seek to prove yourself wrong! (wait, what?)
Anywho, I don't think you need the objContainer variable; it seems to be adding more variables (literally and figuratively) which is making things a little more complicated. And while you can use a function, I don't think you necessarily need one - a simple for loop should suffice. You'll want to declare a variable for the message you're outputting (you can initialize it as an empty string since we'll be doing string concatenation with it later), declare a variable for the student, that will represent each individual student object as we iterate through the array with our for loop.
So, so far we're at
var students = [
{
name: "Cris A.",
track: "Full-Stack Javascript Developer",
achievements: 500,
points: 50000
},
{
name: "Dave M.",
track: "Full-Stack Javascript Developer",
achievements: 230,
points: 77469
},
{
name: "Dylan I.",
track: "iOS Developer",
achievements: 346,
points: 35035
},
{
name: "Bucky R.",
track: "Python Developer",
achievements: 325,
points: 35416
},
{ name: "MPJ", track: "PHP Developer", achievements: 456, points: 42167 }
];
var message = "";
var student;
Your print() function is perfect, so with that, we are here.
var students = [
{
name: "Cris A.",
track: "Full-Stack Javascript Developer",
achievements: 500,
points: 50000
},
{
name: "Dave M.",
track: "Full-Stack Javascript Developer",
achievements: 230,
points: 77469
},
{
name: "Dylan I.",
track: "iOS Developer",
achievements: 346,
points: 35035
},
{
name: "Bucky R.",
track: "Python Developer",
achievements: 325,
points: 35416
},
{ name: "MPJ", track: "PHP Developer", achievements: 456, points: 42167 }
];
var message = "";
var student;
function print(message) {
var divOutput = document.getElementById('output');
divOutput.innerHTML = message;
}
Now, the for loop. The first question should be, "How many times do I want to go through the loop?" To get that answer you should first ask yourself what you are looping through. We're looping through the "students" array because it contains the data that we're trying to display. So we want our loop to stop once we've reached the length of the array because after that there's nothing to loop through.
So, we'll start building our loop.
for (declare/initialize counter variable; counter variable < length of our array; increase the count of our variable) {
//code to execute goes here
}
//which translate to
for (let i = 0; i < students.length; i++) {
//code to execute goes here
}
We initialize "i" to 0 because, if you remember, the first element of an array is at index 0, the second is at index 1, and so on. So our first, student object will be at students[0], which brings us into the for loop.
for (let i = 0; i < students.length; i++) {
//we set our student variable to the index that our counter variable is at in "students"
student = students[i];
//then, since "student" now holds our object, we can access each specific property in our object and use them to construct our HTML
message += `<h2>Student: ` + student.name + `</h2>`;
}
Now all you have to do is follow that same pattern for each property (but use <p>'s instead of <h2>'s for "track", "achievements", and "points") and once you have each property added to message, call your print function (outside of the loop) and pass in your message variable as an argument.
Hope that wasn't too long winded, just wanted to make sure to explain each step. Here's my finished product.

CRIS ACOSTA
2,121 Pointsthanks man!
this would have been a simple solution but I tend overthink a lot and at the same i am confused what Dave was asking.
i was doing a little experimenting and I realized that I can also use the 'for-in' loop as well:
var student;
for(obj in students){
// pass the value of students[obj] to var students
student = students[obj];
//execute code
}
I don't even know why I looped through the object array twice. lol.
thanks again man.

Teresa Balistreri
6,714 PointsWhaaaat??? A brother from a different mother!!! Awesome!

Tammi Carter
5,360 PointsThis was my solution https://w.trhou.se/e2gndi807d
Maya Husic
13,488 PointsMaya Husic
13,488 PointsI actually admire the commitment, you made it your own and the process probably led you to read and learn more. :)