Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial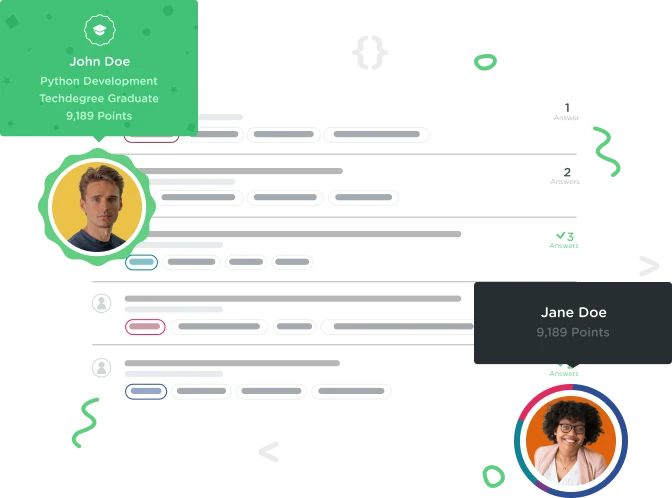

Jiho Song
16,469 PointsWhat about next try syntax?
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
// Connect to the API URL (https://teamtreehouse.com/username.json)
// Read the data
// Parse the data
// Print the data
const https = require('https');
function printMessage(username, badgeCount, points) {
const message = ` ${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript `;
console.log(message);
}
function getProfile(username) {
try {
const request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
let body = "";
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
try{
const profile = JSON.parse(body);
printMessage(username, profile.badges.length, profile.points.JavaScript);
} catch(error) {
console.error(error.message)}
});
});
request.on('error', error => console.error(`Problem with requrest ${error.message}`));
}catch (error) {console.error(error.message);
}
}
const users = process.argv.slice(2);
users.forEach(getProfile);
as you can see the code above, there are two different try and catch, which i expected it to perform individually, however it works only once with first one. Does try and catch only works one time with the first catching and ignoring with further catching?
I'm bit confused with try and catch
1 Answer

Craig Peckett
10,531 PointsWhen the code throws an error it will stop running, therefore it will only log first error it comes across. That way you can go and fix the error and run your code again fixing one problem at a time instead of trying to do multiple errors at once.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 Pointsfixed code formatting