Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial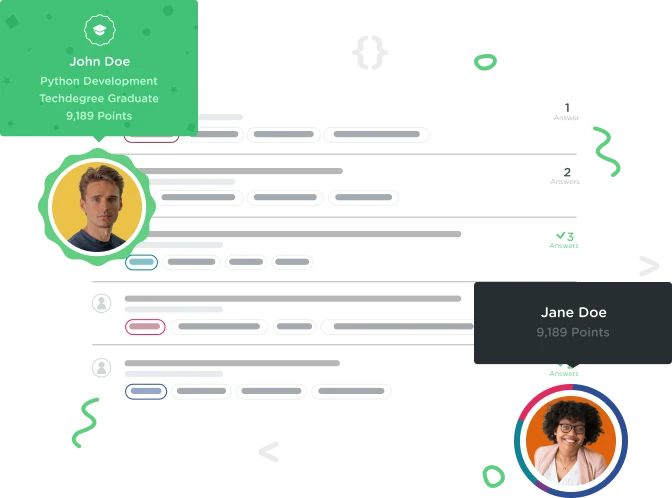
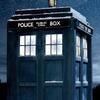
Jaskirat Singh
1,652 PointsWhat am I doing wrong?
// THE FIZZBUZZ GENERATOR!!!!!!
import UIKit
var num = 30
if num % 3 == 0{ println("Fizz") } else if num % 5 == 0{ println("Buzz") } else if (num % 3 == 0) && (num % 5 == 0){ println("FizzBuzz") } else { print ("num") }
What am I doing wrong? I DON'T GET IT!!!!!
3 Answers
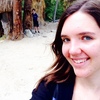
Lauren Hibbs
1,872 PointsGood job, you are on the right track. If you haven't figured it out yet, there are a few things you need to do. -First of all, you will need a for loop or a while loop to iterate through some numbers, unless you only want to test the number 30. -For your last else case, you want to print out what the variable num represents. If you use quotes, you are telling the compiler to print the letters "num", not the variable num.
- Lastly, you probably want to include your "FizzBuzz" if statement first. Otherwise, "FizzBuzz" will never be printed out, because it is in an else if statement. Take the number 15. It is divisible by 3, so code in the first statement will be executed and Fizz will be printed out, and all of the else-if statements will be bypassed. Fizzbuzz will never be printed out.
Here's code that works.
var num = 30
for num in 1...30{ if (num % 3 == 0) && (num % 5 == 0){ println("FizzBuzz")} if num % 3 == 0{ println("Fizz") } else if num % 5 == 0{ println("Buzz") } else { println(num) } }
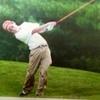
kjvswift93
13,515 PointsYou guys are close. First, there is no need to declare separate variable as you have done with "var num = 30". This is taken care for you when you write "for num in". Here is the answer using your chosen variable name "num"
for num in 1...20 {
if (num % 3 == 0) && (num % 5 == 0) {
println("Fizzbuzz")
} else if (num % 3 == 0) {
println("Fizz")
} else if (num % 5 == 0) {
println("Buzz")
} else {
println(num)
}
}

xavier baril
7,052 PointsHi guys In your first line of code Jaskirat Singh, you didn't use a FOR IN Loop, if you don't want to use this Loop it's possible you can use these lines of code (this code is running well):
var number = 15
if number % 3 == 0 && number % 5 == 0{
println("FizzBuzz")
} else if number % 3 == 0 {
println("Fizz")
} else if number % 5 == 0 {
println("Buzz")
}else {
println(number)
}
this code allow you to be free to change your variable at your own will, and you are not force to use a range of numbers
I hope you get help and the people to come on this page

Robert Smith
2,144 PointsThis is how I did my code, but I became stuck and am wondering if you could help. I did not set %3 == to anything and I am wondering why that is wrong? What does setting %3 == 0 have to do with this?
var number = 25
if number % 3 { println("Fizz") } else if number % 5 { println("Buzz")

xavier baril
7,052 Points% it's mean remainder ; in previous courses Amit gave you some exemple to use it. Remainder allow you to divided two numbers and to observe the Remainder of the division. let's take an exemple : 25 / 5 = 5 remainder 0 25 % 5 = 0 when a number is divided by an other the remainder = 0
That why we use this operator in this case :
numbers % 3 == 0 && numbers % 5 == 0