Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial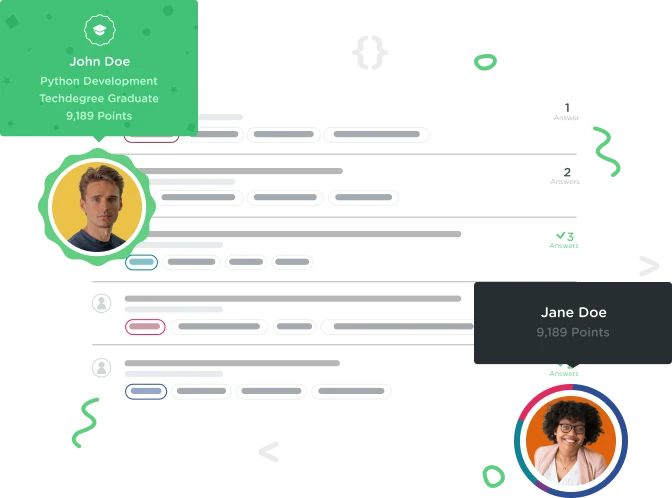

Adam Hoffman
1,813 PointsWhat am i doing wrong ???
I'm stuck in the java validation code challenge could you please tell me what i am doing wrong????
her's my code:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
char [] myArray = fieldName.toCharArray();
if(myArray[0] != 'm'){
throw new IllegalArgumentException ("The first letter must be an m !!!!");
}else{
if(myArray[1] == myArray[1].toUpperCase()){
return fieldName;
}else{
throw new IllegalArgumentException ("The second letter must be uppercase !!!!");
}
}
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
}
}
my error console tells me this error:
./TeacherAssistant.java:8: error: char cannot be dereferenced if(myArray[1] == myArray[1].toUpperCase()){ ^ 1 error
sorry i don't no how to add a picture ;(
Moderator Edited to add markdown to the code
4 Answers
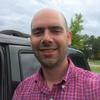
Brandon Adams
10,325 PointsI think you have to use .equals() instead of == in the code. For the != use something like if(!x.equals(y))
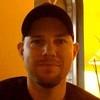
Jeremy Hill
29,567 Pointsit should be something like this:
if((!fieldName.charAt(0).equals('m')) || Character.isLowerCase(fieldName.charAt(1)))
throw new IllegalArgumentException ("Field Name is invalid!");
else
return fieldName;
I had to edit my code- I didn't realize at first that it said that it had to have an 'm' at the beginning.
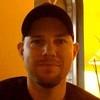
Jeremy Hill
29,567 PointsI had to open my workspace to check it: the code will work like this:
public class javaExample {
public static String validatedFieldName(String fieldName) {
if((fieldName.charAt(0) != 'm') || Character.isLowerCase(fieldName.charAt(1)))
throw new IllegalArgumentException ("Field Name is invalid!");
else
return fieldName;
}
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
}

Martin Krstevski
5,171 PointsIn the if statement where you check if the letter is upper cased you should state as Character.toUpperCase(myArray[1]) It should look like this if(myArray[1] == Character.toUpperCase(myArray[1]))