Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial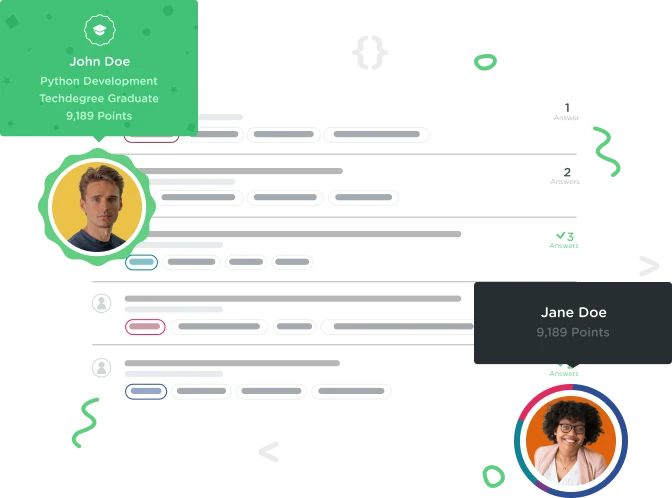

Alex Popian
977 PointsWhat am I doing wrong?
I have no idea what I'm doing wrong and it's killing me. Can someone please tell me what's wrong with my code? Thanks
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public String getTileCount(char amount){
String count ="";
for (char tile : mHand.toCharArray()) {
char display = '-';
if (mHand.indexOf(tile) > -1){
display = tile;
}
count += display;
}
return count;
}
}
1 Answer

Simon Coates
28,694 PointsGoing from memory, maybe something like :
public int getTileCount(char tile){
int count = 0;
for(char letter: mHand.toCharArray()){
if(letter == tile){
count++;
}
}
return count;
}
you don't want to use indexOf, unless you include a starting at parameter. You certainly shouldn't be looking for a character extracted from a string in the same string.
As a demo of indexOf, you might be able to do something similar to.
public int getTileCount(char tile){
int count = 0;
int startAt = 0;
while(mHand.indexOf(tile, startAt) > -1){
count++;
startAt = mHand.indexOf(tile, startAt) +1 ; // update so next loop starts further along the string.
}
return count;
}
(this probably requires a condition to make stop if startAt exceed mhand.length() - 1.)
Alex Popian
977 PointsAlex Popian
977 PointsI don't understand why you can't say: for(char tile: mHand.toCharArray()){ instead of: for(char letter: mHand.toCharArray()){
Simon Coates
28,694 PointsSimon Coates
28,694 Pointsyou can name parameters and variables what ever you want. That's not the problem. The problem is that the method is meant to receive a character, see how many times that character features in the mHand string and return an integer. You seem to be doing something else entirely. You're testing that character features in the string that you got the character from (always true), while not using the amount parameter.