Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial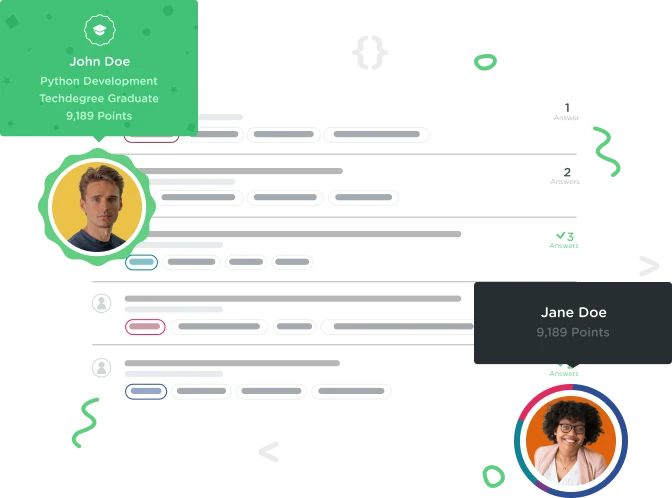

Chigozie Ofodike
11,197 PointsWhat am I doing wrong?
I've initialized this, but for some reason it's saying im still using the member wise initializer.
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(alpha: Double, blue: Double, green: Double, red: Double, description: String) {
self.alpha = alpha
self.blue = blue
self.green = green
self.red = red
self.description = description
}
// Add your code below
}
4 Answers

Alexandre Attar
10,354 PointsFirst you don't need to add a description parameter in you init method. Since we will be assigning the formatted string value directly yo self.description. If we leave the description parameter in our init method and pass it to self.description, as you did before, once you call the RGBColor method it will ask you for a String value for you description parameter, which we don't need in this case. Therefore, it should something like this:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
Hope it helps :)
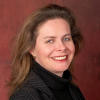
Niki Molnar
25,698 PointsThe challenge asks for the description property to be output in the format "red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0", so you need the self.description to be as follows:
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"

Chigozie Ofodike
11,197 PointsI actually tried this and it said i was using the member wise initializer.

Alexandre Attar
10,354 PointsYeah the issue here is the order of the parameters in the init method.
I edited the answer above, it should work now! If you have any questions let me know :)

Chigozie Ofodike
11,197 PointsSo the order of the parameters has to match how the the member properties were declared?

Alexandre Attar
10,354 PointsTechnically speaking it doesn't have to be, but for clarity purposes it is preferred. You can simply test that on you end by switching the parameters positions in your init method.
For example, this code below will return the same description String as the code above. Since, the values entered will be assigned, just like they were before, to the respective colour constant (red, green, blue, alpha).
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(alpha: Double, red: Double, green: Double, blue: Double) {
self.alpha = alpha
self.green = green
self.blue = blue
self.red = red
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
As long as the values are assign to the correct constant of the struct the order doesn't really make a difference in this case. Especially, since we simply used String Interpolation to construct our description String.
However, on treehouse it does not let you pass the challenge if you don't put them in the same order as they are declared in your struct (At least for this Challenge). We can cal this a bug or they simply force us to write more clearly/efficiently :P
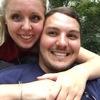
Nicholas Richardson
4,867 PointsFull answers is below, seems like the code checker thing is very sensitive to white space on this one.
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
Chigozie Ofodike
11,197 PointsChigozie Ofodike
11,197 PointsHey, thanks so much! But i tried this and it's giving me an error. When i check what kinda error it is, it doesnt say. Could it be a glitch?