Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial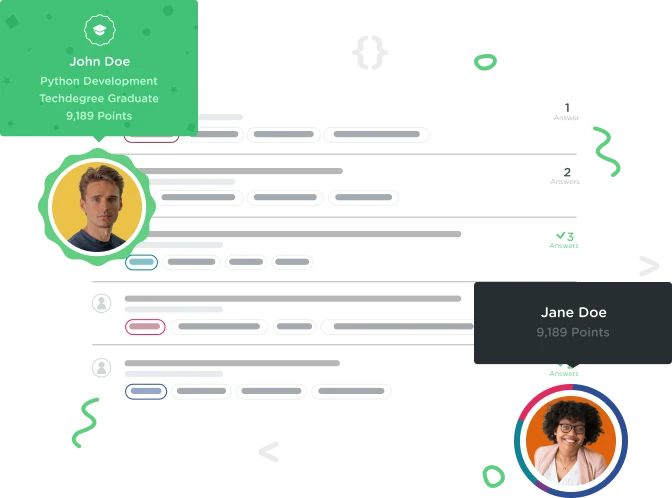

Christopher Ingram
Courses Plus Student 1,977 Pointswhat am I doing wrong?
I keep getting syntax error but I'm not really sure why
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
int lapAmount = lapsDriven + laps;
barCount -= laps;
if (lapAmount > barCount)
throw new IllegalArgumentException ("Not enough battery");
}
lapsDriven = lapAmount;
}
3 Answers
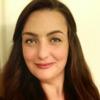
Jennifer Nordell
Treehouse TeacherHi there! You're actually doing really well. There is a problem with your syntax and a slight misplacement of one line. The reason you're getting a syntax error is because you omitted a set of curly braces at your if
statement which results in the lapsDriven = lapsAmount;
line being outside the scope of the method, which means that it's no longer defined. Also, the exception should be thrown before variables have their values changed. Take a look:
public void drive(int laps) {
int lapAmount = lapsDriven + laps;
if (lapAmount > barCount){ // check to make sure we're not trying to run more laps than we have energy bars for
throw new IllegalArgumentException ("Not enough battery"); // If we are, issue this exception
}
// If the amount of laps we want to drive are possible, change the values of these
barCount -= laps;
lapsDriven = lapAmount;
}
Hope this helps!

Lukas Grimm-Strele
793 PointsI was just about to ask a question about this task because i kept getting an error. This was the code I wrote:
public void drive(int laps) {
int newBarCount = barCount -= laps;
if (newBarCount<0) {
throw new IllegalArgumentException("You have to charge");
}
barCount = newBarCount;
lapsDriven += laps;
}
In my opinion my code is better than the one provided from Jennifer Nordell because the Go Kart could be charged between two laps and could drive way more then 8 laps. Either way i think you should include my solution so that people dont get an error message any more. I was starting to loose my mind because I was sure that my solutin would work.
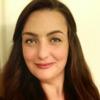
Jennifer Nordell
Treehouse TeacherHi, Lukas Grimm-Strele! I took the liberty of changing your comment to an answer so that it will be eligible for voting.
On a side note, the solution I posted wasn't my solution to the problem. I was simply answering the original OP as to why they were experiencing a syntax error.
In fact, this was my solution, which in my opinion is better than both answers as it doesn't require the allocation of an unnecessary variable and is much more readable.
public void drive(int laps) {
if(laps > barCount) {
throw new IllegalArgumentException("Not enough battery");
}
lapsDriven += laps;
barCount -= laps;
}
}

Lukas Grimm-Strele
793 PointsHi Jennifer Nordell thanks for the quick reply. I am sorry I didnt mean to offend you or disrespect the solution you posted. And you are right, your own solution much better then mine and the other one posted. Will the programm accept your answer as correct or does it only accept the first answer?
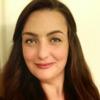
Jennifer Nordell
Treehouse TeacherYes, my solution passes . And no offense taken! I just wanted to make it clear that I do, on occasion, help others to make their solutions work for them even if it isn't necessarily the approach I would take personally. One of the beautiful things about programming is that there is very often more than one way to solve any given problem!