Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial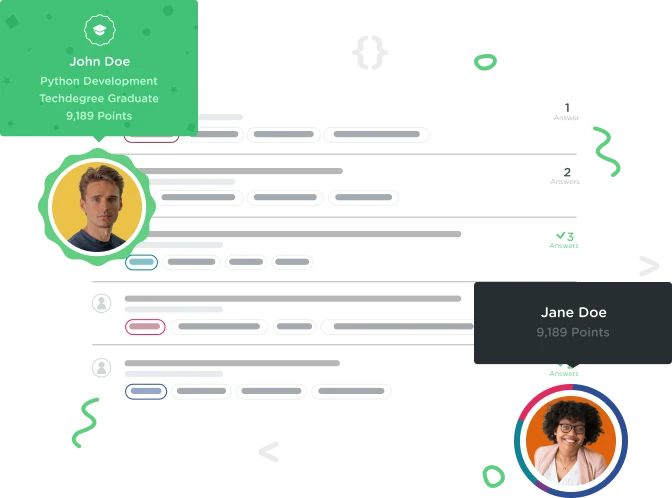
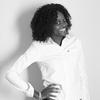
Nomzamo Sithole
61,838 PointsWhat am I doing wrong?
Now I need you to write a function named absolute that takes two arguments, a path string and a root string. If the path is not already absolute, return the path with the root prepended to it.
For example: absolute("projects/python_basics/", "/") would return "/projects/python_basics/" while absolute("/home/kenneth/django", "C:\") would return "/home/kenneth/django".
>>>> import os
>>>> os.path.absolute("projects/python_basics/", "C:/home/kenneth/django")
2 Answers
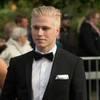
Johan Rönkkö
28,054 PointsYes you have to. Ok, so I will walk you through my solution.
def absolute(path, root):
if(not os.path.isabs(path)):
new_path = ''.join((root, path))
return new_path
else:
return path
I define the function "absolute" that takes two arguments - a path and a root (since POSIX and Windows use different root-signatures). I then check if the path that was passed in is NOT an absolute path. I do this with the "abs" method from the "os.path" package. If it's not an absolute path, I create a new variable that will hold the value of the path prepended with the root (I do this with the built in join function on a empty string), and then i return the variable. However, if the path is an absolute path, i.e. if the IF-statement returns False, then I just return the path that was passed in as it is.
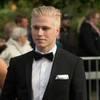
Johan Rönkkö
28,054 PointsImport os and create a function that takes two arguments like so
import os
def absolute(path, root):
pass
Now use the isabs method from os.path and check if the path that was passed in is NOT an absolute path. If it's not, prepend the root-argument to the path and return the new path (with pythons join-method for instance). Else return the path as it is.
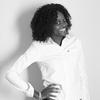
Nomzamo Sithole
61,838 PointsThank you for response. i want to know if i have to put it in an IF else statement, to determine the result wanted?
import os
def absolute(path, root):
pass
os.path.isabs("/home/kenneth/django")
os.path.isabs("projects/python_basics/")
os.path.join("projects/python_basics/", "/")
Nomzamo Sithole
61,838 PointsNomzamo Sithole
61,838 PointsThank you Johan,
Now I understand, just didnt know how to implement it