Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial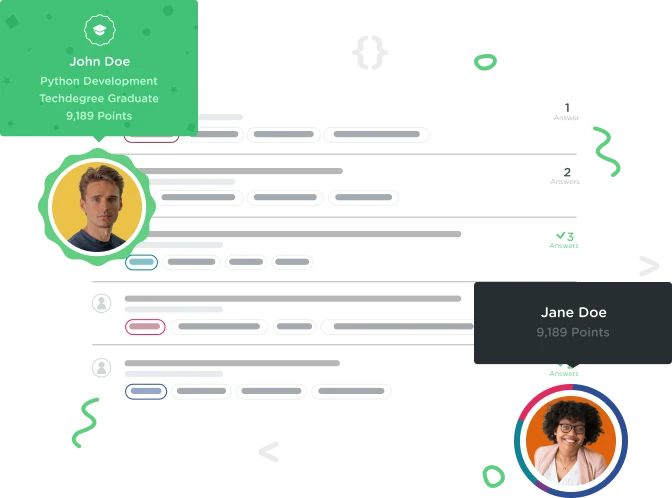
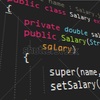
simon123123
1,291 PointsWhat am I doing wrong
public class SignUpActivity extends Activity {
public static final String TAG = SignUpActivity.class.getSimpleName();
protected SignUpCallback mSignUpCallback = new SignUpCallback() {
@Override
public void done(ParseException e) {
}
};
// onCreate() and other code has been omitted
protected void signUp(String username, String password) {
ParseUser newUser = new ParseUser();
newUser.setUsername(username);
newUser.setPassword(password);
newUser.signUpInBackground(new SignUpCallback(){
@Override
public void done(ParseException e) {
if (e == null) {
}
else {
Log.e(TAG, "ERROR");
}
}
});
}
3 Answers

Joe Steele
10,721 PointsHey Simeon Gellert, I'm not sure what error you are running into, but there are 2 things I noticed in your code.
You define your SignUpCallback
, mSignUpCallback
, and then don't actually use it, but instead pass a separate, new SignUpCallback
to the newUser.signUpInBackground
function.
Also, inside of both of the signup callbacks, you don't actually do anything when the signup is done. (i.e. if (e == null)
)
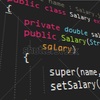
simon123123
1,291 Pointsprotected void signUp(String username, String password) {
ParseUser newUser = new ParseUser();
newUser.setUsername(username);
newUser.setPassword(password);
newUser.signUpInBackground(new mSignUpCallback(){
@Override
public void done(ParseException e) {
if (e == null) {
}
else {
Log.e(TAG, "ERROR");
}
}
});
}
}
this is the error I get when I change it to mSignUpCallback
./SignUpActivity.java:24: cannot find symbol symbol : class mSignUpCallback location: class SignUpActivity newUser.signUpInBackground(new mSignUpCallback(){ ^ 1 error

Joe Steele
10,721 PointsmSignUpCallback
is an instance variable that's already defined there at the top of the file. You wouldn't want to instantiate like you would a class.
So in other words, you had:
newUser.signUpInBackground(new SignUpCallback() {
// your done function
});
or in the case of this latest comment...
newUser.signUpInBackground(new mSignUpCallback() {
// your done function
});
And you'll want to change that to
newUser.signUpInBackground(mSignUpCallback);
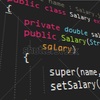
simon123123
1,291 PointsSo like this?
import android.app.Activity;
import android.util.Log;
import com.parse.ParseUser;
import com.parse.ParseException;
import com.parse.SignUpCallback;
public class SignUpActivity extends Activity {
public static final String TAG = SignUpActivity.class.getSimpleName();
protected SignUpCallback mSignUpCallback = new SignUpCallback() {
@Override
public void done(ParseException e) {
}
};
// onCreate() and other code has been omitted
protected void signUp(String username, String password) {
ParseUser newUser = new ParseUser();
newUser.setUsername(username);
newUser.setPassword(password);
newUser.signUpInBackground(new SignUpCallback);
@Override
public void done(ParseException e) {
if (e == null) {
}
else {
Log.e(TAG, "ERROR");
}
}
});
}

Ben Jakuben
Treehouse TeacherJoe has done a great job explaining the problem and solution. You don't need to use this code:
newUser.signUpInBackground(new SignUpCallback);
@Override
public void done(ParseException e) {
if (e == null) {
}
else {
Log.e(TAG, "ERROR");
}
}
That's recreating the SignUpCallback that's already defined as mSignUpCallback above. This code challenge is teaching you how to pass in callback methods as parameters instead of declaring new callbacks each time. So get rid of that code, then instead pass it as a parameter like Joe shows:
newUser.signUpInBackground(mSignUpCallback);
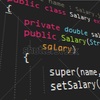
simon123123
1,291 PointsThanks I finally made it :)
simon123123
1,291 Pointssimon123123
1,291 PointsHey Joe, I'm not sure exactly what you mean. Can you show me what the finished code should look like? This is the error I'm running into "Make sure you use the 'Log.e()' method to log the message from the instructions!"
Thanks :)
Joe Steele
10,721 PointsJoe Steele
10,721 PointsAh, sorry! I forgot to ask which step of the challenge you were on. Based on your reply, it looks like you are task 3 of the challenge.
In task 2 however, the challenge asks you to pass the
mSignUpCallback
callback that was initialized at the top into thenewUser
'ssignUpInBackground
function. In your version of the code you are passing a brand new SignUpCallback instead of using the one at the top. This appears to still work for task 2, but will cause problems on task 3 due to how we check the challenge for validity.If you pass the
mSignUpCallback
to the function and do your error checking andLog.e(TAG, "ERROR");
inside of that callback, it should then work.As a side note, Android's
Log.e()
function has another variant that allows you to pass the exception as the third argument. I noticed that this version will not work with this code challenge and will say there was a compiler error. That's due to how we are testing for this particular challenge, so just use theLog.e(TAG, "ERROR");
, 2 argument version of the function for this challenge like you are already doing.Joe Steele
10,721 PointsJoe Steele
10,721 PointsSee also this related post for an example of what I described above.