Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial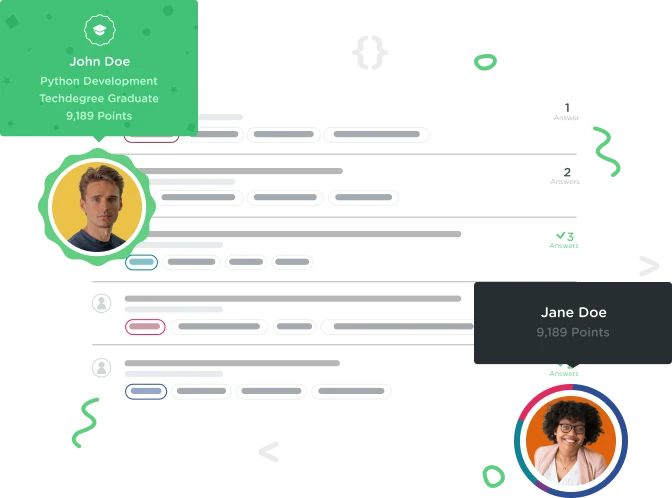

John Gilmer
Courses Plus Student 3,782 PointsWhat am I doing wrong?
Can't figure out what is wrong with this.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(location: Location) {
}
}
let Buisness = someBusiness(name:"Google", location: 12)
1 Answer

andren
28,558 PointsThere are a number of issues:
You need to define a
name
parameter for theinit
method, not justlocation
You need to set the
name
andlocation
properties equal to the parameters passed in within theinit
method.When creating an instance you need to name the constant
someBusiness
and callBusiness
, not the other way around.The
location
parameter expects an instance of theLocation
struct, which12
is not.
Here is the solution with some comments added:
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
// Define init method with name and location as parameters
init(name: String, location: Location) {
// Set the name property equal to the name parameter
self.name = name
// Set the location property equal to the location parameter
self.location = location
}
}
// Create a Business instance by passing in a string and an instance of the Location struct
// and assign it to a constant named someBusiness
let someBusiness = Business(name:"Google", location: Location(latitude: 0.00, longitude: 0.00))
kelly jewkes
1,765 Pointskelly jewkes
1,765 PointsThe added comments really help. Thank you.