Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial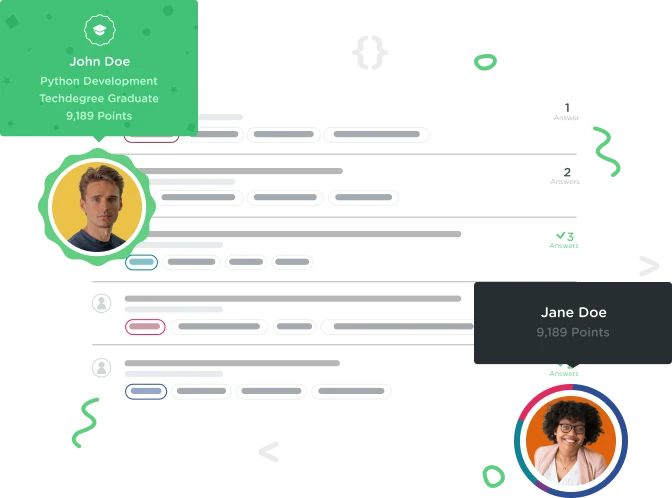
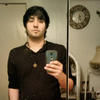
William Sanders
11,396 PointsWhat am I doing wrong?
namespace Treehouse.CodeChallenges { class Frog { public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
bool EatFly(int distanceToFly){
if(distanceToFly <= tongueLength)
{
return true;
}
else {
return false;
}
}
}
}
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
bool EatFly(int distanceToFly){
if(distanceToFly <= tongueLength)
{
return true;
}
else {
return false;
}
}
}
}
2 Answers

Tobias Karlsson
2,751 PointsYou are missing the visibility keyword public
in the method definition and you are referring to the wrong property tongueLength
with a lowercase "t". It should be an uppercase "T".
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(int distanceToFly)
{
if (distanceToFly <= TongueLength)
{
return true;
}
else
{
return false;
}
}
}
}
Also, you do not need the if-statement at all in the EatFly method. The if condition can be returned directly because it evaluates to either true or false like so:
public bool EatFly(int distanceToFly)
{
return distanceToFly <= TongueLength;
}
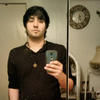
William Sanders
11,396 PointsThank you! I figured it out and forgot about this post!!