Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial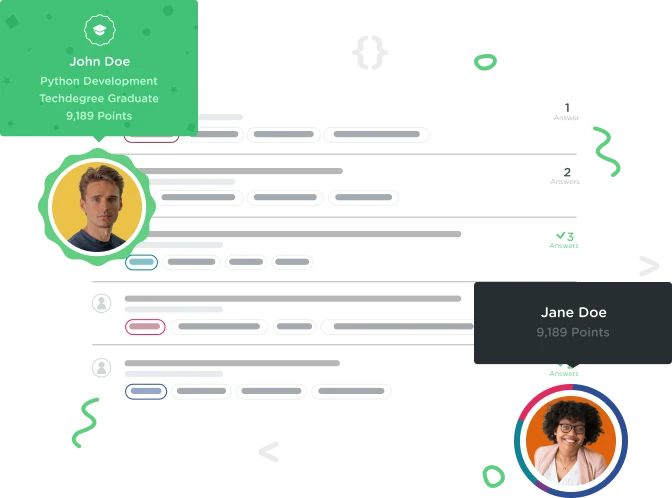

Sergei Kobyakov
397 PointsWhat am I doing wrong?
What am I doing wrong?
// Enter your code below
let value = 200
let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15
let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below
let result:Int = value % divisor
// Task 2 - Enter your code below
if (result == 0) {
let isPerfectMultiple:Int = result
}
1 Answer
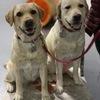
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Sergei,
I can understand how this challenge might be confusing. The challenge text is somewhat vague about what it wants you to end up with, and the error text is misleading because the code you wrote does compile.
Let's get a handle on what the question really wants from you, and then we can look at your code to see why it isn't successful.
Your step 1 code is fine, but when we get to step 2, the challenge asks:
Compare the value of
result
to 0 using the equality operator and assign the resulting value to a constant namedisPerfectMultiple
.
So the question wants there to be a constant with the name isPerfectMultiple
at the end of your code. Because you declare your constant inside the if
statement, you never end your program with a value in isPerfectMultiple
(it disappears at the end of your if
statement). I believe this is what is causing a compiler error: somewhere Treehouse is adding code to your code that looks for isPerfectMultiple
, but it doesn't exist.
Even if isPerfectMultiple
did persist beyond your if
statement, hopefully you can see that it would only ever have a value if the if
test evaluated to true
(if you're not seeing that right away, mentally step through your code in the situation where divisor
does not perfectly divide into value
(e.g., 7 and 8).
So, if you want to lay out your code the way that you have, then you need to declare (but not initialise) isPerfectMultiple
before your if statement, then assign a value to it inside your if statement (making sure to also cover the circumstance where result
is false
) (use else
).
Then your code will have a value for isPerfectMultiple
at the end of your program and you will no longer have a compiler error.
However, you will still have an error because what the question wants isPerfectMultiple
to be is the result of comparing result
to 0 (i.e., true
or false
), not the value inside result
(i.e., an int
). So instead of assigning result
to isPerfectMultiple
, you assign true
when your if
evaluates to true
or false
when your if
evaluates to false
.
At this point, you might be thinking, 'it seems very long-winded to have to write an if and an else just to assign the result of a true/false comparison into a variable.` And you would be right. While the above-described fixes will cause your code to pass the challenge, there is a much better way.
It comes from remembering that result == 0
is not just a test, it is a value, either true
or false
and like any other value, it can be assigned to a variable or constant. Consider the following example:
let a = 3
let b = 5
let a_is_greater_than_b = a > b
print(a_is_greater_than_b) // will print 'false'
Hope that clears everything up
Cheers
Alex