Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial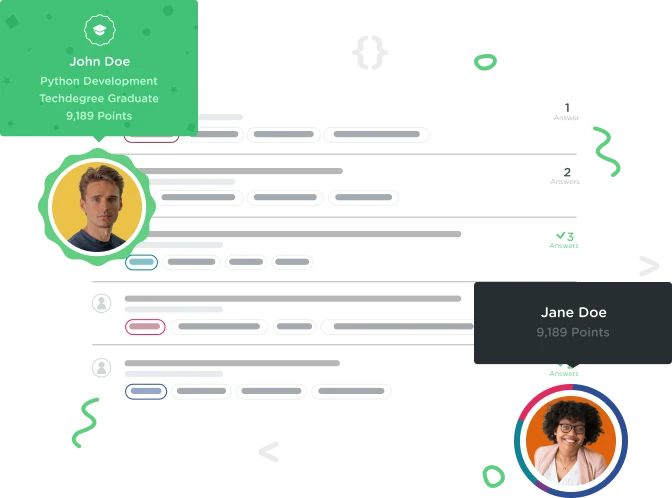

Dylan Chuckry
2,841 PointsWhat am I doing wrong?
I might be drunk, help please :(
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
messy_list.insert(0, messy_list.pop(3))
fixed_list = []
for each in messy_list:
if each == int:
fixed_list.append(each)
else:
continue
messy_list = fixed_list
3 Answers
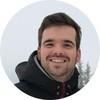
John Lack-Wilson
8,181 PointsHey Dylan, thanks for your question.
There are loads of ways to do this, although I quite like your approach because it is very explicit in what you are trying to do. The problem is related to your conditional for checking if each == int. You want to instead check the type of element in the messy_list array. See here for details about the type() builtin.
Here's a simple example:
an_integer = 1
if type(an_integer) is int:
print("An Integer!")

Dylan Chuckry
2,841 PointsThank you for the tip John! 'type()' seems quite useful. Would you mind giving me a quick explanation as to why using the '==' comparison doesn't word the same way?
I have ran into a new problem now though.
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
messy_list.insert(0, messy_list.pop(3))
messy_list_copy = messy_list
for each in messy_list_copy:
if type(each) is not int:
messy_list.remove(each)
It returns the integers as well as the list. I assume this is because the data types inside of the list are all ints as well. I know I can solve it by creating another if statement and removing list data types but I am wondering of there is a more proper way of solving it?
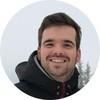
John Lack-Wilson
8,181 PointsGreat question! I may not be 100% correct with what I am saying here, but as far as I'm aware, the reason why if each == int doesn't work is because it is making the comparison between a reference i.e. the each part and a type i.e. the int part. The actual value that the reference is referring to may be an int, however the reference is not. That's the key thing to remember here, is that variables are simply referencing something, they are not the actual value or type. I hope that is both correct and makes sense!
The new issue seems to be related to checking if it is not an int. If you go back to your previous approach where you are appending to a new empty list if it is an int, then that should pass the challenge for you.

Dylan Chuckry
2,841 PointsAh, that makes sense thank you. And yes I was doing that but the challenge requires you to use del or .remove.
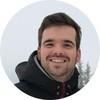
John Lack-Wilson
8,181 PointsAhh yes you're right! I'd forgotten the challenge does specify that (although it does pass without using either of those functions).
If you'd like to pass the challenge by using .remove() or del, then you should use list.copy(messy_list) rather than assigning messy_list_copy to messy_list. The difference is when you say messy_list_copy = messy_list, you are assigning them to the exact same list. This has issues when you are iterating over the list because any changes you make are reflected on both messy_list and messy_list_copy. Whereas using messy_list_copy = list.copy(messy_list), you are making a copy of the list and then assigning it to that. Any changes made to one of the lists will not change the other.