Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial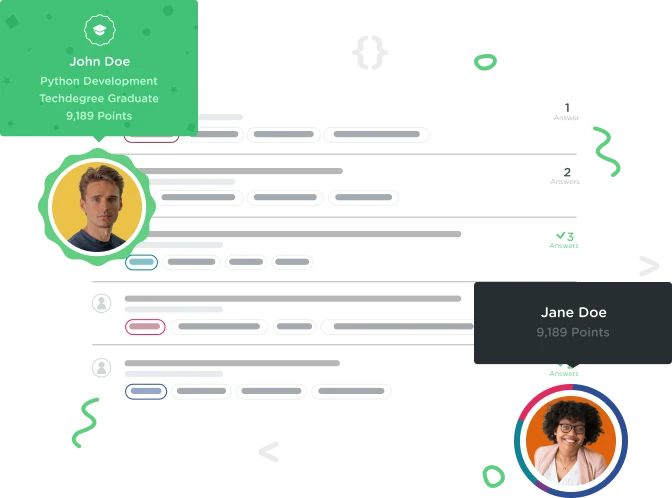

Christopher Flores
6,898 PointsWhat am I doing wrong?
I know this is an odd set of code, but I'm only writing it this way to complete the challenge and move on.
At this point, since I keep get the answer wrong I'm just writing whatever I can for the echo variable to see if I can eventually get it right. Doing it so many times just seems to fry my brain a little.
Can anybody spot the flaw? It's probably something VERY small that I'm not noticing
*To clarify, the challenge keeps saying my echo variable is incorrect and that it's not syncing up with the "return"...
function returnValue(argument) {
var value = argument;
var echo = argument + value + returnValue;
return value;
}
returnValue('what is the value of your argument?');
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
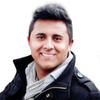
Camilo Lucero
27,692 PointsYou seem a bit confused on how functions work, consider rewatching that section. Better to learn slow but solid, than to try to go as fast as you can.
The challenge asks you to create a function named returnValue that just returns the argument it is passed in, so you declare the function with just one parameter and then inside the body of the function, return the parameter:
function returnValue(argument) {
return argument;
}
The next part is to create a variable called echo and store the value that the function returns. So, in the next line we'll create the variable and set it equal to the function and pass it any string we want:
var echo = returnValue('Hi there');
Now echo will be equal to 'Hi there', because it is the value that the function returns.

Emmanuel C
10,636 PointsCreate a function that takes a argument and returns it immediatly
function returnValue(argument){
return argument;
}
Create a variable named echo AFTER your function that holds what gets returned when you call your function. It takes any string as an aurgument
function returnValue(argument){
return argument;
}
var echo = returnValue('This string gets returned from returnValue and stored in echo');

Christopher Flores
6,898 PointsThanks guys,
You both helped equally. I suppose I need to take these challenges slower, or hopefully retain the lessons more.
The biggest thing that tripped me up was putting the variables inside of the function's { } - because I thought I had to. I appreciate your answers :)