Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial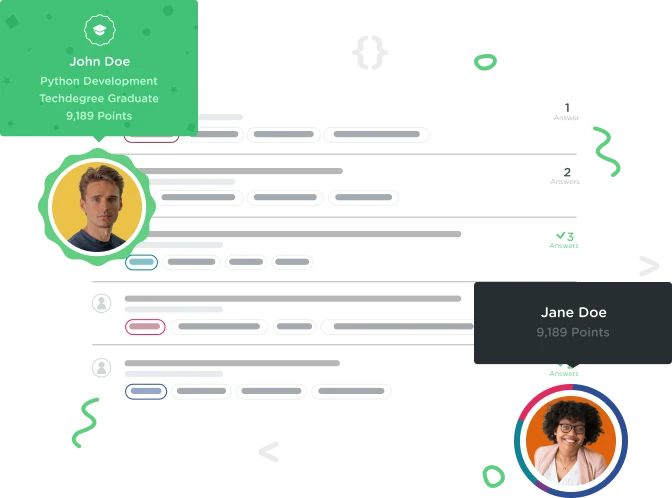

S.D. Willems
310 Pointswhat am i doing wrong? error code is: Does your GetVideoGames doesn't have any parameters
""" public VideoGame GetVideoGames(int id){
VideoGame gamee = null;
foreach(var game in _videoGames){
if(game.Id == id)
gamee = game;
break;
}
return gamee;
}
"""
using Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
// TODO Add GetVideoGames method
// TODO Add GetVideoGame method
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
public VideoGame GetVideoGames(int id){
VideoGame gamee = null;
foreach(var game in _videoGames){
if(game.Id == id)
gamee = game;
break;
}
return gamee;
}
}
}
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
2 Answers
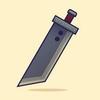
Allan Clark
10,810 PointsYou are a little ahead of yourself. The first challenge asks you to create a GetVideoGames method that accepts no parameter and returns the private field. What you have is trying to answer the second challenge. So the first challenge should look like this.
public VideoGame[] GetVideoGames() {
return _videoGames;
}
As for the second challenge you are pretty close. Make sure you adjust the name of the method, it should be GetVideoGame (singular bc we are returning only one). My suggestion would be to just remove the 'break' from the loop entirely. It is generally considered good practice to avoid keywords such as break, continue, and goto that manually manipulate the execution flow of the code. This is because it can lead to unforeseen and hard to diagnose bugs in your code. As written the method will go into the foreach loop, check the id of the first element of the array and then break out of the loop, never getting the chance to check the other elements.
Simply removing the break line will fix this issue and will pass the second challenge, but since there are use cases where breaking would make sense this is what the code would need to look like: (Note: this would only be used if you are searching through a very large array probably ~10k+, and at that point there are other optimizations that would be needed as well)
public VideoGame GetVideoGame(int id){
VideoGame gotGame = null;
foreach(var game in _videoGames)
if(game.Id == id) {
gotGame = game;
break;
}
return gotGame;
}
Let me know if you have any more questions, and keep up the great work! Happy Coding!! :)

S.D. Willems
310 PointsGreat explanation! Thanks!