Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial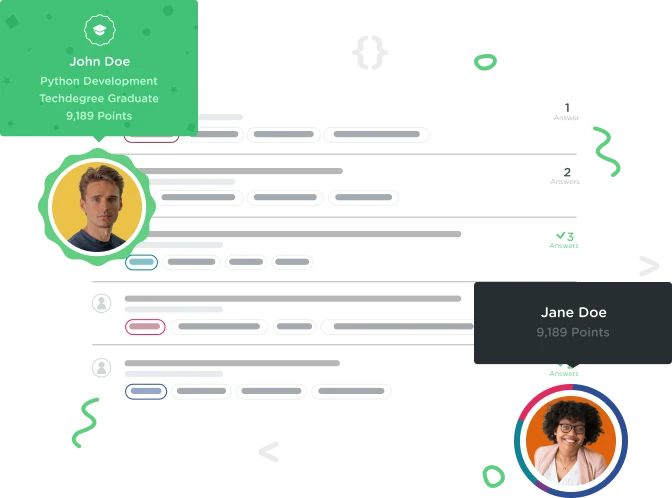

Arthur Kakulidis
2,657 PointsWhat am I doing wrong here?
I don't know how I'm getting this wrong. Someone please help, thanks!
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
numbers.forEach( number => times5.push(numbers * 5));
// times5 should be: [5,10,15,20,25,30,35,40,45,50]
// Write your code below
2 Answers
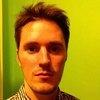
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsYou're very close. You're using numbers
where you should be using number
:
numbers.forEach( number => times5.push(number * 5));
The forEach
method is going to:
- loop through each item in the array
- invoke a callback that you pass in
- pass the item as an argument into that callback.
So in this case, we're passing in an anonymous arrow function: number => times5.push(number * 5)
. number
is the parameter we're giving that function, and then using in the body of that function. It might clarify something if we define that function somewhere else and then pass it in as the argument to forEach
:
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
function multiplyByFiveAddToList(num) {
times5.push(num * 5)
}
numbers.forEach(multiplyByFiveAddToList);
So in the example above I'm defining a new function multiplyByFiveAddToList
. Then when I call forEach
, it takes a function as an argument, I pass in the function I defined called multiplyByFiveAddToList
. Then, it will loop through each item in the array and call that function, passing in the item.
forEach
is an example of a higher order function. They're a tricky concept to wrap your head around. But it will help you understand what's going on when you're written your own higher order functions, not just using higher order functions that come automatically with JavaScript.
There are a few other courses at Treehouse that might help you with this topic:
Practice ForEach in JavaScript
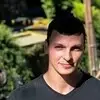
Kallil Belmonte
35,561 PointsYour mistake is here:
times5.push(numbers * 5)
You are multiplying numbers * 5, and the correct should be number * 5.
So the correct anwers is this:
numbers.forEach( number => times5.push(number * 5));
More about the forEach method: https://www.w3schools.com/jsref/jsref_foreach.asp

Arthur Kakulidis
2,657 PointsThank you so much! I understand it now.
Arthur Kakulidis
2,657 PointsArthur Kakulidis
2,657 PointsAlso, how does Javascript know what the parameter "number" is, if you didn't assign any arguments to it?