Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial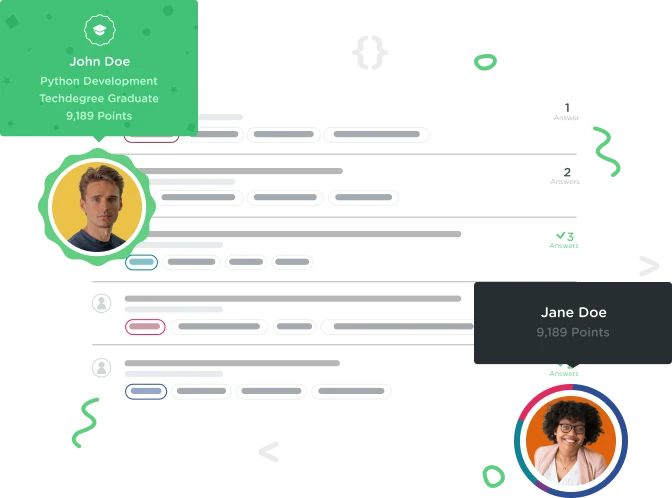
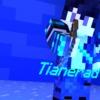
Tianerad 999
4,063 PointsWhat am I doing wrong here?
Task:
Okay, so I need to count how many occurrences of a a specific letter is in the player's tiles. Let's build that over a couple of steps. I've added some example use cases in Example.java
Create a new method named getCountOfLetter that returns an int, and requires a parameter of type char named letter. For this task, just make it return 0.
I have written the code down below, I believe I got the if statement wrong in the tiles.toCharArray.
I have also tried replacing
return progress;
with
return 0;
and
return "0";
Please answer back!
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter() {
int progress = "";
for (char letter : tiles.toCharArray()) {
char display = '-;
if (tiles.indexOf(letter) != -1 {
display = tile;
}
}
return progress;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
2 Answers

scribbles
21,173 PointsLoop over the tiles as a character array (as you are doing) and check to see if each tile is equal to the argument passed to the method (which you forgot to include):
public int getCountOfLetter(char letter) {
int count = 0;
for (char tile : tiles.toCharArray()) {
if (tile == letter) {
count++;
}
}
return count;
}
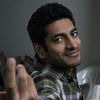
Priyajot Syan
Courses Plus Student 2,643 PointsData types are very important. And understanding what can be stored in what is important. In getCountOfLetter you are intializing int to "" which means you are trying to convert int to String in wrong way.
You can convert string to Integer using Wrapper classes but i dont think that is part of the current scope.
A few other mistakes i can see is if (tiles.indexOf(letter) != -1 , no closing bracket char display = '-;, missing closing symbol
And scribbles said above increment your count when you find a match
int indexOf(int ch): It returns the index of the first occurrence of character ch in a given String
IndexOf Char will always return the first ocurrence so i dont think we can use this here. So even if you have two 'd''s it will match just with first 'd' and return true
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherAre you on/asking about Task 1 or 2 in this challenge?