Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial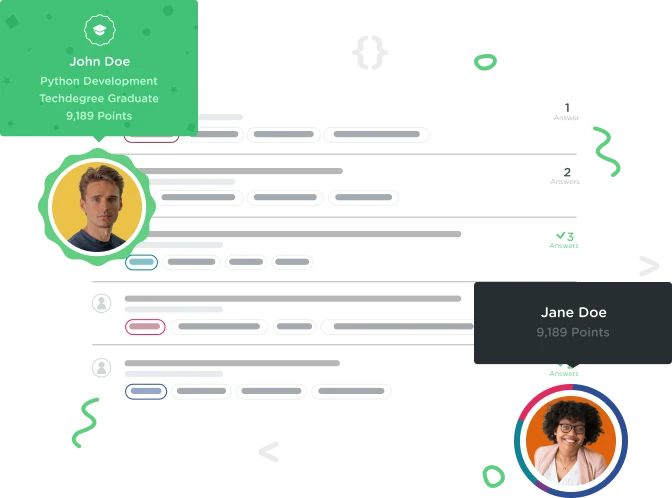
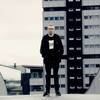
Kyle Bolton
2,493 PointsWhat am I doing wrong here?
Think I'm doing everything correct here but get a 'code took too long' to run error. Any help?
var continueToPrompt = true;
var secret = prompt("What is the secret password?");
while(continueToPrompt) {
if(secret !== "sesame"){
continueToPrompt = false;
}
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
4 Answers

Roberto Alicata
Courses Plus Student 39,959 PointsYou need to put the comparison in the while condition and the prompt into the while loop:
var secret = "" ;
while( secret !== "sesame" ){
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");

Tyler Haas
20,623 Pointsyou need to move the secret variable down into the while loop so that it prompts the user to enter another secret password. right now you have an endless loop as once the password matches there is nothing to break it out of the loop. also I think you want to test if the password entered === "sesame".
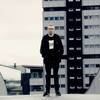
Kyle Bolton
2,493 PointsCould you provide the right code? Tried your explanation but is asking me to use "!==" instead of "===".
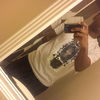
Michael Singleton
6,253 Pointsvar secret = "sesame"; // this sets your secret password.
var guess; // this will hold the user's guesses
//this loop will ask the user for input until their input matches secret while (guess !== secret) { guess = prompt("What is the secret password?"); // here you're just re-setting the guess variable with the user's input }
//we've now exited the loop, which means the user gave us the right input (the secret password) document.write("You know the secret password. Welcome.");

Roberto Alicata
Courses Plus Student 39,959 PointsIt just start the while loop testing the variable secret. The first time secret is equal to "" so the test returns False and the program enter the loop. In the loop the variable secret is associated to the returning value of the prompt and then it tests again for the while loop.
Kyle Bolton
2,493 PointsKyle Bolton
2,493 PointsCould you explain further with this? Still struggling to make heads or tails of this.