Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial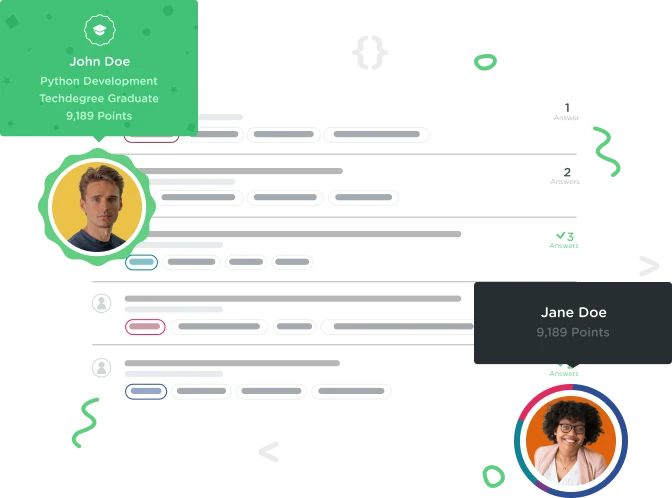
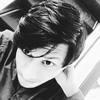
Mit Sengupta
13,823 PointsWhat am i doing wrong here?
class Car: Vehicle { var numberOfSeats: Int
init(numberOfSeats: Int) {
self.numberOfSeats = numberOfSeats
}
}
let someCar = Car(numberOfSeats: 4)
2 Answers
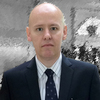
Nathan Tallack
22,159 PointsYou were very close. But you forgot that you still have to satisfy the parent class init parameters when you create the Car object. Also, as a shortcut, you do not need to write an initializer for your subclass if you are setting the default values for the properties when you declare them. Consider the following code.
class Car: Vehicle {
var numberOfSeats: Int = 4
}
let someCar = Car(withDoors: 4, andWheels: 4)
Note we did not need to put the parameter in for numberOfSeats when creating the Car object because we are letting the default value of 4 be used. We could have specified it if we wanted a different value though.
While not part of this challenge, consider if you did not have a default value and did need to write an init for the subclass. Always remember you need to call the parent class init from your subclass following your init, so that the properties of that class can be initialized also. Consider the following code.
class Car: Vehicle {
var numberOfSeats: Int
init(withDoors doors: Int, andWheels wheels: Int, withSeats seats: Int) {
self.numberOfSeats = seats
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 4, andWheels: 4, withSeats: 4)
Note here we passed the parameter for the seats in and that we call the parent class init method with the super.init after our own parameter assignments in our subclass init.
I hope this helps. :)
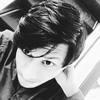
Mit Sengupta
13,823 PointsThank you Nathan, I understand it now. :) Very helpful!
Mit Sengupta
13,823 PointsMit Sengupta
13,823 PointsThank you so much for taking the time to explain it to me. :) Can you please explain when should I use override init instead of just init in a subclass?
Nathan Tallack
22,159 PointsNathan Tallack
22,159 PointsSometimes you want to override a method of a parent class in a subclass. Say your parent class had a method called description and you wanted to have a method of the same name in your subclass that is specific to that subclass. You would declare it with the override keyword to show the compiler you are not unintentionally overriding a method you did not know existed in your parent class.
The init method is a little different for overrides. Consider the following code.
Here our subclass is using override on its custom designated initializer. It is doing it because it has no scope local properties of its own to initialize and calls the parent designated initializer using the same parameters () with super.init so that it can get the inherited variable property from its parent class before it modifies the property value.
So in this case we are using override on an init method because this initializer matches the same as the parent initializer (which is a default initializer because its properties are declared with initial values).
Sorry, I know it does not read well. I was quite lost with all of this the first handful of times I attempted to understand it. But do let us know if you are still having trouble following and I or others here on the forum will be happy to answer any of your questions. :)