Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial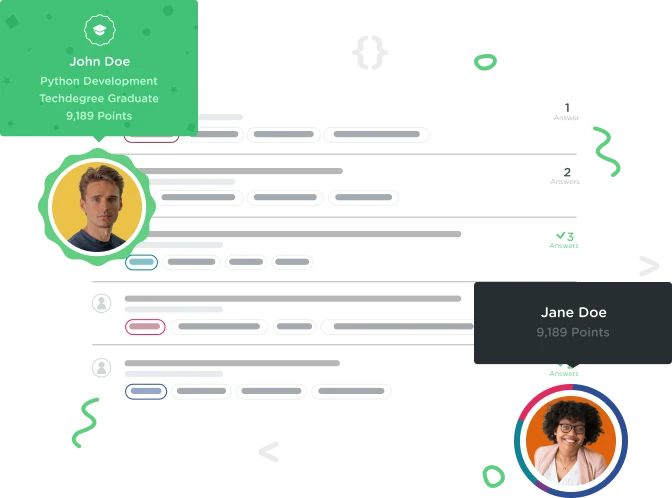

blako heaud
140 PointsWhat am I doing wrong here?
task is to create a method named charge that sets the new barCount field to the maximum amount of bars available for each GoKart.
class GoKart {
public int barCount;
public static final int MAX_BARS = 8;
private String color;
public GoKart(String color) {
this.color = color;
}
public charge void() {
barCount = MAX_BARS;
}
public String getColor() {
return color;
}
}
3 Answers

blako heaud
140 PointsIt still does not work :( can you type how should i write that method?

Daniel Marin
8,021 PointsThe answer is pretty straight forward. So the charge method is void and you simply assign barCount to MAX_BARS
public void charge() {
this.barCount = MAX_BARS;
}
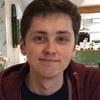
Stephen McBride
7,769 PointsHey Blako, I think you have two problems with you code that I can see.
1) You have set you field barCount as public when it should be private in the challenge. It should be like so:
private int barCount;
2) You have set your return type the wrong way round, with void after the method name. It should be the method type and then the method name. It should be like so:
public void charge() {
barCount = MAX_BARS;
}
This is my code for reference:
class GoKart {
private int barCount;
public static final int MAX_BARS = 8;
private String color;
public GoKart(String color) {
this.color = color;
}
public void charge() {
barCount = MAX_BARS;
}
public String getColor() {
return color;
}
}
I hope this helped solve your problem.