Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial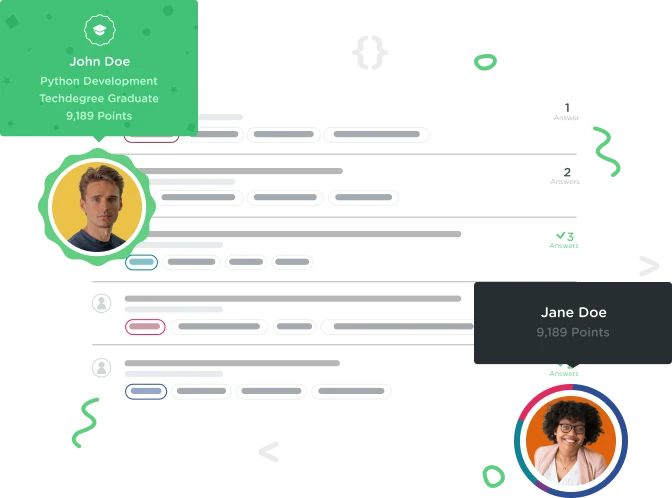
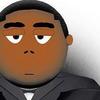
Joshua Howard
12,798 PointsWhat am I doing wrong here?
I see that this can be done in three lines of code. I guess i'll make get to the point where I can do this w/ my eyes closed in the fewest lines possible. But as of now, I don't see why this isn't working (even though not the most efficient way).
def sillycase(blueball):
da_length = len(blueball)
half_length = da_length / 2
firstHalf = blueball[:half_length]
secondHalf = blueball[half_length:]
secondHalf = secondHalf.upper
return int(firstHalf) + int(secondHalf)
2 Answers
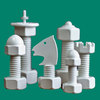
Steven Parker
231,269 PointsYou got pretty close, but there were four issues remaining:
-
half_length = da_length // 2
"You'll want to use the int() function or integer division, //"
-
firstHalf = firstHalf.lower()
(new line added) "the first half should be lowercased"
-
secondHalf = secondHalf.upper()
method calls need parentheses after the name
-
return firstHalf + secondHalf
keep the strings as strings (no "int" conversion)
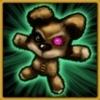
Dave Harker
Courses Plus Student 15,510 PointsHi Joshua Howard,
It's a good effort, however there are a few things to consider:
- .upper() is a string method, you'll need the ()
- you might want to add a .lower() to your firstHalf as there is no guarantee it will be lowercase already
- you'll need to fix that return statement so it returns a string that is made up of firstHalf + lastHalf
I think you'll be fine to get it working now. Keep going, you're almost there!
Happy coding,
Dave.
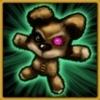
Dave Harker
Courses Plus Student 15,510 PointsJust one other thing ... reading your post again I note you state:
I see that this can be done in three lines of code
You could do it in a single return statement if you really wanted to but while that might be true it would mean bunching up a couple of method calls directly in the return statement. So yeah, a little less code, it's also a lot less readable.
Efficiency is obviously important, but so is readability and while there is room to improve in what you've got, don't be too hard on yourself :) That was a quality attempt you made there.
Also, do you really want to read a return line that looks like this (not meant to be efficient - 2x len calc - but ... single line):
return someWord[:(int(len(someWord)/2))].lower() + someWord[(int(len(someWord)/2)):].upper()