Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial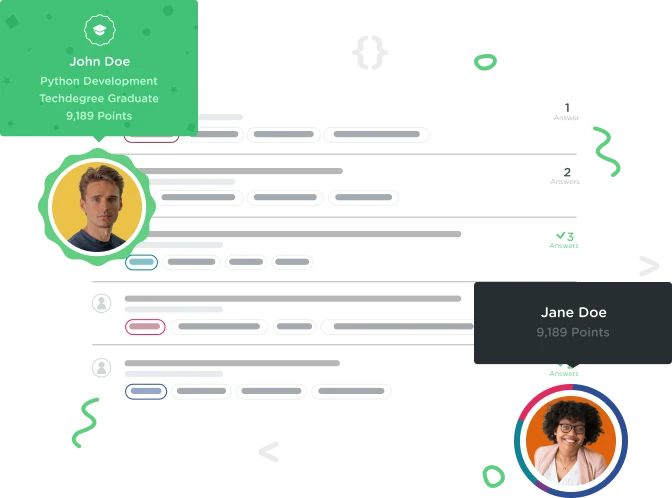
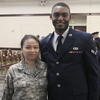
Darrin Spell Jr
Full Stack JavaScript Techdegree Student 10,303 PointsWhat am I doing wrong here?
What exactly am I doing wrong here? Can someone explain please.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
pattern = [".", "_"]
for pat in pattern:
if pat == ".":
self.pattern.append("dot")
elif pat == "_":
self.pattern.append("dash")
return "-".join(pattern)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
1 Answer
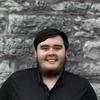
Michael Hulet
47,912 PointsYou have a couple issues here, but you're overall pretty close.
First of all, your return
statement is indented to be inside your for
loop. Thus, no matter how many elements are in self.pattern
, your loop will always stop and your function will return
after analyzing just the first element. You can mitigate this by unindenting that return
statement a level, so it's even with your for
loop
Second, you're creating a new list called pattern
inside your __str__
function with some elements in it, looping over that, and append
ing your results to self.pattern
, which is an entirely different list. Instead, you should be creating a new, empty list (which you can name how you please), looping over self.pattern
, and append
ing the result to the new list you created at the beginning of the __str__
function. At the end of the function after the loop, you can return
that array, and you should be good to go
After you fix those 2 issues, your code passes the challenge on my machine. Great job!
Darrin Spell Jr
Full Stack JavaScript Techdegree Student 10,303 PointsDarrin Spell Jr
Full Stack JavaScript Techdegree Student 10,303 PointsI don't understand what you mean by appending the results to self.pattern. Do I do that on another line? Would I do self.append?
Michael Hulet
47,912 PointsMichael Hulet
47,912 PointsNope, I'm referring to the 2 lines where you have
self.pattern.append
. Instead, you should be appending to a new list that you created at the beginning of the functionDarrin Spell Jr
Full Stack JavaScript Techdegree Student 10,303 PointsDarrin Spell Jr
Full Stack JavaScript Techdegree Student 10,303 PointsSomething like this
Michael Hulet
47,912 PointsMichael Hulet
47,912 PointsNot quite. In your code
patterns
is an entirely different list fromself.patterns
. I'd name it something different so that's more clear tbh. You want to iterate overself.patterns
and only append to your localpatterns
(which I recommend you rename). You'll also have tojoin
your localpatterns
when youreturn
, too, and notself.patterns
. You can think ofself.patterns
as being your input andpatterns
being your outputIt looks like you have your
return
indented properly now, however. You're almost there!