Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial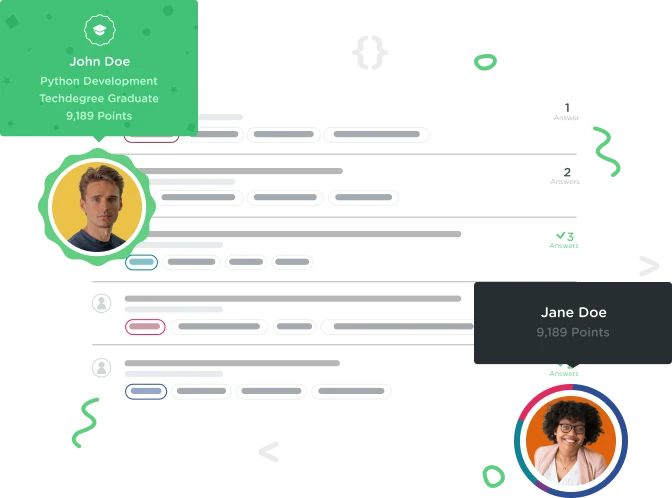
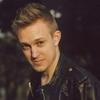
Johnny Linnert
3,239 PointsWhat am I doing wrong here? All seems fine when I run this in Playgrounds.
I've added a method named description to the struct Post. At the bottom, I've called the instance method from firstPost and assigned it to the constant postDescription.
In Playgrounds I get the result that Treehouse is asking for, but when I submit it to the Treehouse interface it says I've made a mistake.
What am I doing wrong?
struct Tag {
let name: String
}
let tagName = Tag(name: "swift")
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tagName.name)"
}
}
let firstPost = Post(title: "iOS Development", author: "Apple", tag: tagName)
let postDescription = firstPost.description()
1 Answer

Anjali Pasupathy
28,883 PointsYou're nearly there! In your description method, you need to change "tagName.name" to "tag.name". You should be referencing the tag that's a part of your Post struct ("tag"), not the tag you instantiated ("tagName").
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
I hope this helps!
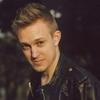
Johnny Linnert
3,239 PointsThanks so much, Anjali and Kyle -- really appreciate your help here.
Yeah, as it turned out, the exercise didn't explicitly state that I needed to create an instance of Tag
but I created one anyway. hehe

Anjali Pasupathy
28,883 PointsYou're welcome!
Whether you create an instance of Tag and store it in its own constant, or create an instance of Tag when you're instantiating firstPost, doesn't matter for this task; the only thing they require is that you initialize a Post with an instance of Tag. I've seen people write perfectly valid code in which they initialize a Post with a String for the name property of Tag, and create an instance of Tag in their init method. The code functions perfectly fine, but the quiz compiler doesn't like it because they're not passing an instance of Tag into their init method.
Kyle Lambert
1,969 PointsKyle Lambert
1,969 PointsHi Johnny, I hope this helps.
Its almost right... Just need to change a couple of things. First of all we don't need to declare a constant of Tag and set is equal to "swift". When we create an instance in the second to last line of code, we set the value for 'tag' here, so it can we altered accordingly. And secondly just change the function return slightly as well as the firstPost tag instance.
It should look like this,