Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial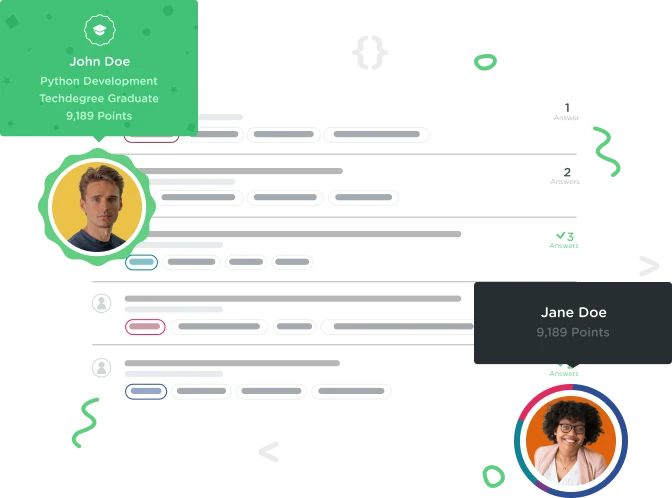

Vaibhav Tank
3,129 PointsWhat am I doing wrong here? It keeps telling me to "instantiate the contents of the dictionary from the plist!."
Here is my code:
import Foundation
// Add your code below
let plistPath = NSBundle.mainBundle().pathForResource("CrazyInformation", ofType: "plist")
let dictionary = NSDictionary(contentsOfFile: plistPath)
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsThe problem here is that pathForResource
returns an optional String -> String?
, as it might well be that the file does not exist/can't be found. However, you do not unwrap the return value in your code. In practice, you would be doing something like this
// Optional binding
if let plistPath = NSBundle.mainBundle().pathForResource("CrazyInformation", ofType: "plist") {
let dictionary = NSDictionary(contentsOfFile: plistPath)
}
or
// Optional binding
if let unwrappedPlistPath = plistPath {
let dictionary = NSDictionary(contentsOfFile: unwrappedPlistPath)
}
For the sake of this course, you may also force-unwrap the return value. This is, of course, not a good idea in practice, as you can never be 100% sure that the return value is not nil.
// Forced unwrapping
let dictionary = NSDictionary(contentsOfFile: plistPath!)