Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial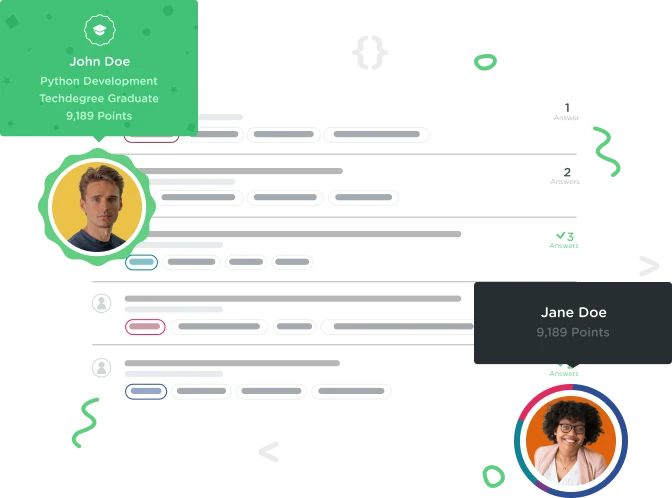
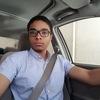
Luqman Shah
3,016 PointsWhat am I doing wrong? I'm also not really understanding this question.
I'm not understanding this question, what it's asking me to do, is something we did not go over in the previous video, I think. I don't know how to execute this code!
for ( counter = 0; counter < 156; counter +=1 ) {
console.log(counter);
}
console.log(counter);
EDIT:
Ok so I re-attempted this and passed the quiz challenge, this is what I did:
for ( counter = 4; counter < 156; counter +=1 ) {
console.log(counter);
}
console.log(counter);
Someone please explain this to me xD I sort of get it, still not too sure though. So basically they just want you to add 156 numbers to the console, just starting from the number 4?

K Cleveland
21,839 PointsSo this is what your code is actually doing:
//starting at 4 (this is our starting variable and value)
for ( counter = 4;
//as long as counter is less than 156 (this is the "exit" condition)
counter < 156;
//add 1 to counter (this won't change the original value until after it's been evaluated, so counter will remain 4)
counter +=1 ){
//then log the value for counter (which is 4)
console.log(counter); //this loop will run and output all numbers from 4 to 155
}
//extra console.log outside of the for loop. This will be 156
console.log(counter);
The way your code is written, you begin at 4 and increment +1 until you reach a point where counter equals 156. At that point, you exit the loop and don't execute any code in the body. You're not adding 156 numbers. You're starting at 4 and logging numbers out until you reach the exit condition, which is counter < 156.
Like this:
counter = 4 (this is less than 156) so log 4 to console
counter = 5 (this is less than 156) so log 5 to console
counter = 6 (this is less than 156) so log 6 to console
counter = 7 (this is less than 156) so log 7 to console
...
counter = 153 (this is less than 156) so log 153 to console
counter = 154 (this is less than 156) so log 154 to console
counter = 155 (this is less than 156) so log 155 to console
counter = 156 (this is NOT less than 156 ) so exit the loop
The way the code is written, what's actually happening is:
4 5 6 ... 155 (exit the for loop) 156
BUT
Even though you passed this challenge, you didn't really do it all in a for-loop. If I remove that extra console.log(counter); that is outside the loop this won't pass, and the reason why is because your loop will exit when counter = 156 and so it will never console.log(counter) when it's 156.
Good luck!
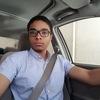
Luqman Shah
3,016 PointsJoel Price & K Cleveland Thank you so much! So basically like you said, Cleveland, I'm not really logging in 156 numbers, I'm just adding one counter to the counter variable until the condition is false, once it is false, it exits the loop, but if there wasn't a log function outside of the loop, you're saying that it won't log the number 155? And basically we're just logging in the counter? Also, why exactly do we have two log functions in this code? one inside the loop and one outside the loop. Please do also read my comment I made on Steven Parker 's asnwer. Also I wish I could give you best answer but your answer is a comment.
1 Answer
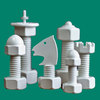
Steven Parker
229,788 PointsAs Keaunna pointed out, you didn't quite implement the loop as expected. If you change your comparison to "less than or equal" (<=), you won't need the extra console.log
statement after the loop and the loop will do everything needed.
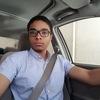
Luqman Shah
3,016 PointsWhy does a change in comparison defeat the purpose of needing an extra log function? All it does is check to see whether counter will ever be less than or equal to 156, thus logging in 156, right?
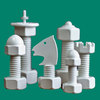
Steven Parker
229,788 PointsI think you answered your own question there. But to illustrate, here's the original code:
for (var counter = 4; counter < 156; counter +=1) {
console.log(counter);
}
console.log(counter);
And here it is with the comparison changed, eliminating the extra line:
for (let counter = 4; counter <= 156; counter +=1) {
console.log(counter);
}
Both do exactly the same thing, but the latter one does it with fewer lines (and is a bit easier to understand on first glance). It also allows the scope of "counter" to be limited to the loop using "let", where the first one only works if it is global.
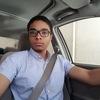
Luqman Shah
3,016 PointsOhh ok I get it, so it's not the comparison difference that defeats the purpose of needing an extra console.log,
it was just never needed to begin with. I was confusing myself but it was just something simple to understand, thank you.
Joel Price
13,711 PointsJoel Price
13,711 PointsThat's exactly right. They want you to log only the numbers 4 to 156. So it makes sense to start at 4 so it doesn't log anything outside of that range. Good job solving it by yourself, btw.