Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial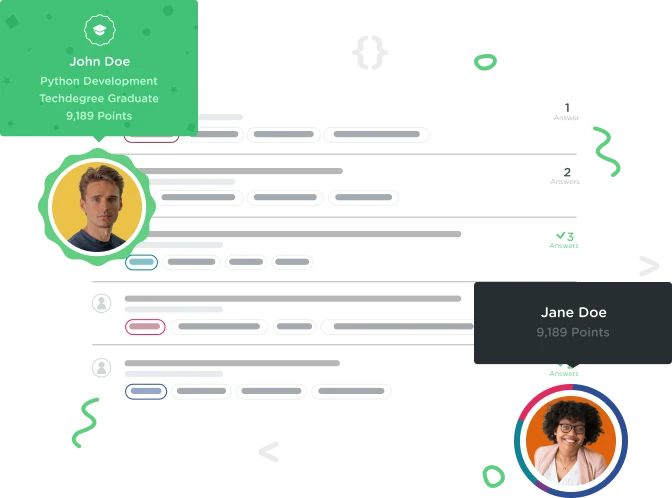

olamilekan oshodi
959 PointsWhat am i doing wrong. My parsefloat not working.
Here is my code.
var name = 'John Smith';
var visitTime = prompt('What time did you see ' + name + ' today?');
var finishTime = prompt('What time did you leave ' + name + ' today?');
var totalTime = parseFloat('visitTime') + parseFloat('finishTime');
document.write('<h3>WoW! Cool you manage to finish seeing ' + name + ' at ' + totalTime);
I need to put in time which i believe will be decimal number. He said i need to put in a quote in order generate decimal number in return for parseFloat.
2 Answers
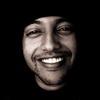
miikis
44,957 PointsIn addition to Carlos Federico Puebla Larregle's answer, in order to subtract dates like that, you would need to use the built-in Date object. In fact, a slightly better way of doing what you're trying to do would be something like this:
var name = 'John Smith';
var visitTime = prompt('What time did you see ' + name + ' today?');
var finishTime = prompt('What time did you leave ' + name + ' today?');
// Subtracting two date objects will return their difference in milliseconds
var totalTimeInMs = new Date(finishTime) - new Date(visitTime);
// Divide milliseconds by 1000 to get seconds
// Divide seconds by 60 to get minutes
// Divide minutes by 60 to get hours passed
var totalHours = totalTimeInMs / 1000 / 60 / 60;
// Then you'll have: "Wow! Cool you manage to finish seeing Wilbur in 5 hours"
document.write('<h3>WoW! Cool you manage to finish seeing ' + name + ' in ' + totalHours + ' hours');
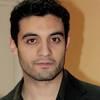
Carlos Federico Puebla Larregle
21,074 PointsIf you're going to put a variable as an argument to the "parseFloat()" function you have to omit the quotation marks. Like this:
var totalTime = parseFloat(visitTime) + parseFloat(finishTime);
I hope that helps a little bit.
olamilekan oshodi
959 Pointsolamilekan oshodi
959 PointsI guess right Mikis. But now am getting "WoW! Cool you manage to finish seeing John Smith in NaN hours" after running your code.
so have done is, i input random number in the first question and second question to the hours in decimal like. 11.00 - 12.30, should give me 1 hour 30 minute.
or i still doing some thing wrong or maybe my code and your code doesn't work for this particular script
thanks
miikis
44,957 Pointsmiikis
44,957 PointsRight. So, for the above to work, visitTime and finishTime must be a certain format. You can read about all the acceptable formats here.
If you don't want to read that and you just want to get it to work, try a format like this, where today's date and time would be: "March 19, 2016 02:33:30pm".
Here, try this: