Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial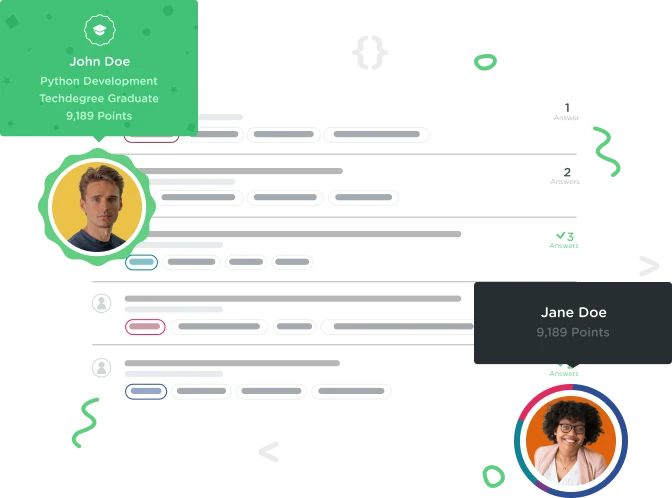

Jackson Monk
4,527 PointsWhat am I doing wrong to convert this?
For this project I need to convert every string value in the numarr array to a number, but my parseInt method isn't working. Can anyone see what my problem is?
<html>
<head></head>
<body>
<script>
window.onload = function() {
let body = document.getElementsByTagName('body')[0];
let input = document.getElementsByTagName('input')[0];
let button = document.getElementsByTagName('button')[0];
let ul = document.getElementsByTagName('ul')[0];
button.addEventListener('click', () => {
let numarr;
let numbers;
let counter = 0;
let valid = true;
let funct;
let text;
let li;
function program() {
numbers = input.value;
numarr = numbers.split(' ');
for (let z = 0; z < numarr.length; z ++) {
parseInt(numarr[z]);
if (isNaN(numarr[z])) {
valid = false;
break;
}
}
if (valid === true) {
sum();
}
else {
alert("Input must be a number. Please try again");
}
function sum() {
for (let a = 0; a < numarr.length; a ++) {
for (let b = a + 1; b < numarr.length; b ++) {
for (let c = b + 1; c < numarr.length; c ++) {
if (numarr[a] + numarr[b] + numarr[c] === 0) {
text = document.createTextNode(numarr[a] + ", " + numarr[b] + ", " + numarr[c]);
li = document.createElement('li');
li.appendChild(text);
ul.appendChild(li);
text = undefined;
li = undefined;
}
}
}
}
}
}
program();
});
body.style.backgroundColor = 'violet';
body.style.color = 'yellow';
input.addEventListener('mouseover', () => {
input.style.backgroundColor = 'lightgray';
});
input.addEventListener('mouseout', () => {
input.style.backgroundColor = 'white';
});
button.style.backgroundColor = 'tomato';
button.style.color = 'orange';
button.style.border = 'tomato';
button.addEventListener('mouseover', () => {
button.style.backgroundColor = 'orange';
button.style.color = 'tomato';
});
button.addEventListener('mouseout', () => {
button.style.backgroundColor = 'tomato';
button.style.color = 'orange';
});
};
</script>
</body>
</html>
2 Answers
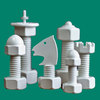
Steven Parker
230,274 PointsThis code is referring to elements with tags like "input", "button" and "ul"; but there's nothing in the body except for the script itself.
Also, "parseInt" doesn't change its argument, it returns a number result. To make use of that result you would need to assign it to a variable or pass it as an argument to a function.

Jackson Monk
4,527 PointsSo you're saying to get the number I'm looking for, I have to assign the parsed values to a variable or pass them to a function? (Just want to be clear)
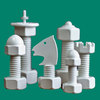
Steven Parker
230,274 PointsFor example, if you wanted to change the the value in the variable from a string to a number:
numarr[z] = parseInt(numarr[z]);
Calling "parseInt" by itself performs the conversion but does not change anything.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsUse the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
 Or watch this video on code formatting.