Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial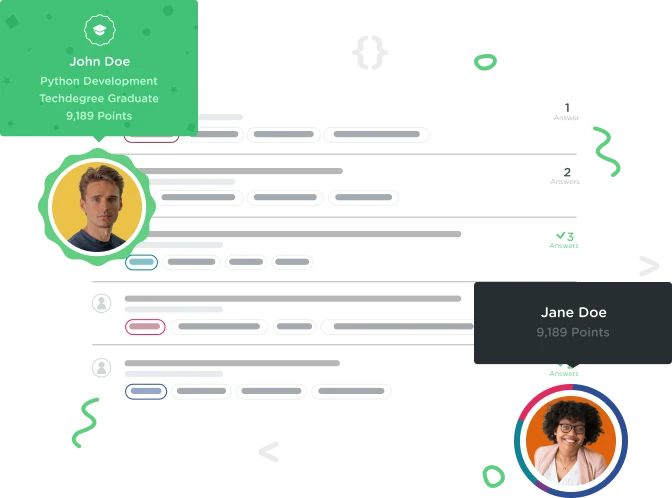

Aaron Fry
826 PointsWhat am I missing?
I have already tested this on other programs and it works. Please advise me if they want it done a certain way because I have asked a fellow friend who codes much bigger things and he doesn't see a problem.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(random_string):
final_dict = {}
string_words = random_string.split(" ")
for item in words:
if item not in word_tracker:
word_tracker[item.lower()] = 1
else:
word_tracker[item.lower()] += 1
return final_dict
2 Answers

Cheo R
37,150 PointsRunning your code, I got the following errors:
NameError: name 'words' is not defined
fixed with changing words to string_words
NameError: name 'word_tracker' is not defined
fixed with word_track = {}
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(random_string):
final_dict = {}
word_tracker = {}
string_words = random_string.split(" ")
for item in string_words:
print("Item is: {}".format(item))
if item not in word_tracker:
print("\t not in word_tracker, adding {}".format(item.lower()))
word_tracker[item.lower()] = 1
print(word_tracker)
else:
print("\tis in word_tracker, updating {}".format(item.lower()))
print(word_tracker[item.lower()])
word_tracker[item.lower()] = word_tracker[item.lower()] + 1
return word_tracker
print(word_count("I do not like it Sam I Am")) # {}
print(word_count("I do not like it Sam I Am") == {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}) # False
Do you see what it's returning? Look at your code and trace it backwards. I added a few print statements, as you see, it never gets to the else branch.
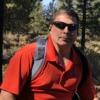
Mark Kastelic
8,147 PointsHi Aaron,
Several things I see here:
- You make a list called string_words and then try to iterate over "words" which is not defined. So, the correct statement should be: for item in string_words:
- You initialize final_dict but then use word_tracker as your dictionary. Replace all references to word_tracker with final_dict.
- When you make the above changes, it runs, but only finds a single instance of each word ( "I" should be found twice). This happens because you don't convert the random_string to lower case first. Instead, you only convert the key of the dictionary element to lower case. So when you iterate through the string_words (which contains capital "I" twice) your logic tests if item not in final_dict (which has all lower case keys), so the else statement is never executed.
Hope this helps!