Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial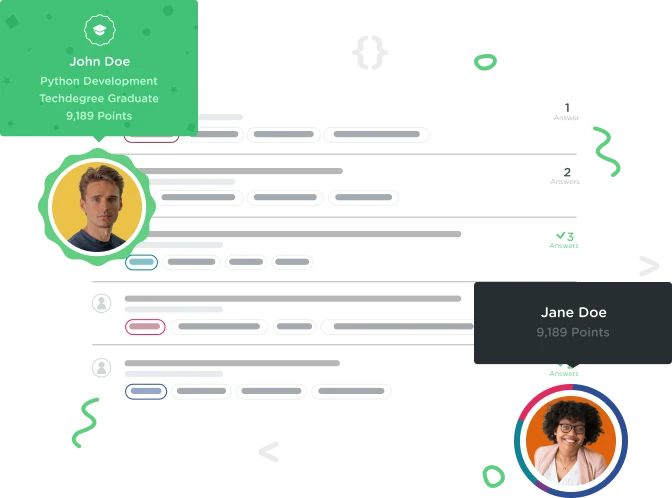

Michael Russell
1,575 PointsWhat Am I Missing?
I started off following the instructions as basic as possible to create the requested "feedback" method:
def feedback(self, grade):
if self.grade > 50:
return self.praise
return self.reassurance
However, I kept getting errors where it said that the value for "grade" was undefined. So my current code looks as it does now, yet I am getting "Exception: EOF" errors apparently. Could someone please explain or give me a hint as to what I'm missing? I would a appreciate it.
Michael
class Student:
name = "Michael"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
self.grade = input("Please enter your grade, {}: ".format(self.name))
self.grade = int(self.grade)
if self.grade > 50:
return self.praise
return self.reassurance
1 Answer
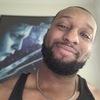
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi Michael,
So I'm first going to attempt to explain why I think you're getting the EOF exception. EOF stands for 'end of file'. But I don't actually think that it's your file that the interpreter is reaching the end of. I imagine that the way treehouse grades your challenges is, they write unit tests that run when you click 'done'. This particular unit test probably declares a variable and stores a new instance of the Student class to it (as an object). Then it probably calls the feedback method on that object, while passing in some integer (hidden from you) as the argument. Then it checks to see if the output is equal to what it should beβgiven the argument passed in. And that's the entire test (I'm guessing, as I don't actually write the tests for treehouse).
Your feedback method requires additional input though. So the unit test runs, and when it's expecting your code to output a string that starts with either "You inspire me..." or "Chin up...", you're asking that the user enter a grade. The test doesn't know what to do with that, because the end of its file has been reached.
Here's the thing though, you don't actually have to ask for additional input. The grade will be passed in when the method is called, as you've made that parameter a requirement. You just have to make sure you use that data, because as of right now you are not. Instead, you're declaring a new grade variable that you're attaching to the instance of the Student, and as mentioned previously, you're requiring input to determine what data that variable holds.
There's two ways you can fix this:
The first is to not use 'self.grade'. Comment out or delete the first two statements in your feedback function, then within your control statement evaluate if grade (not self.grade) is greater than 50.
The other way would be to declare a variable named grade on the instance of the Student, but then assign the value of the grade argument to it (that way you're not asking for additional input).
Once you've got that sorted you're going to run into another issue with the return statements in your feedback method. Here's a hint for you on that: Make sure you're invoking those methods in your return statements.
Michael Russell
1,575 PointsMichael Russell
1,575 PointsThank you for getting back to me, Brandon. When it came to the code for the feedback method, I changed "self.grade" to just "grade", and realized that I hadn't properly invoked the methods in the return statements, because I forgot to include parentheses at the end. After some mild revision, my code for the feedback method looked as follows:
This ended up being the correct solution to the task. Finally understood what I was missing. Thank you once again for your assistance and helpful hints. Take care.
Michael