Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial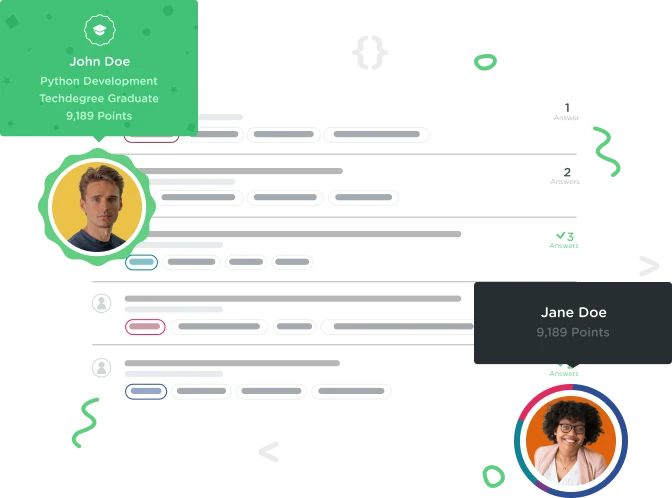
Kathleen Stearns
1,318 PointsWhat am I missing?
I've entered in what I thought was the correct code, and got a syntax error that after (this) the firstName, lastName wasn't needed. When I took it out it said that it was needed. Clearly I'm missing something else, what is it?
function Person(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
Person.prototype.fullName = function() {
return this.firstName + " " + this.lastName;
};
function Teacher(firstName, lastName, roomNumber) {
Person.call(this, firstName, lastName);
this.firstName = firstName;
this.lastName = lastName;
this.room = roomNumber;
}
2 Answers
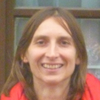
Maja B.
12,984 PointsI think you should take
this.firstName = firstName;
this.lastName = lastName;
out of Teacher constructor.
But inside of a Person constructor they should stay.

kheiferfuller
8,635 PointsYour code is technically correct but try to think of it in terms of DRY coding. You're restating the function in there. When you call
Person.call(this, firstName, lastName);
You're running your code through the Person.js which already contains
this.firstName = firstName;
this.lastName = lastName;
so the editor is seeing that you've both of them and is getting a little peeved, I think. I'm not sure where your error was exactly. But based on your current code, if you were to rewrite your code to demonstrate that you understand the call function as below, it should work just fine.
function Teacher(firstName, lastName, roomNumber) {
Person.call(this, firstName, lastName);
this.room = roomNumber;
Colton Ehrman
Courses Plus Student 5,859 PointsColton Ehrman
Courses Plus Student 5,859 PointsWhen you do
Person.call(this, firstName, lastName)
it will automatically setup
this.firstName = firstName and this.lastName = lastName