Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial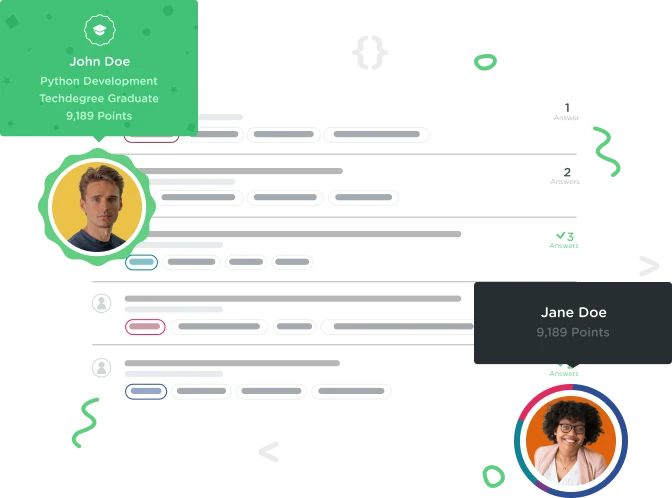

Katherine Lucas
658 PointsWhat am I missing?
It says I have a parse error. Need help please.
var secret;
var correctGuess = false;
do {
secret = prompt('What is the secret password?");
if (secret === 'sesame') {
correctGuess = true;
}
while ( ! correctGuess ) {
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
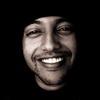
miikis
44,957 PointsHi Katherine,
You're missing a couple things: your do...while syntax is messed up and your prompt question's quotations don't match. Fix those and it runs fine; let me know if it doesn't.
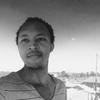
Dan Weru
47,649 PointsHi Katherine, you're doing great just make a few tweaks.
var secret;
var correctGuess;
do {
secret = prompt("What is the secret password?");
if (secret === "sesame") {
correctGuess = true;
}
}while (!correctGuess);
document.write("You know the secret password. Welcome.");
This should work ... be consistent while using double(") or single(') quotation marks.
You must not necessarily set a value(initialise) for the correctGuess variable ... just declaring it with no set value defaults to undefined. You could set it to false though; it does no harm.
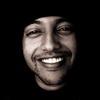
miikis
44,957 PointsYour refactor is wrong — it doesn't make any sense. The goal of refactoring is to optimize for something; what exactly are you optimizing for here?
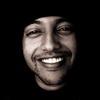
miikis
44,957 PointsAlso, null is not strictly equal to false.
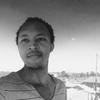
Dan Weru
47,649 PointsHer code was not working in the first place. The document.write function was outside the body of the loop. That means it would be called every time, regardless of the loop. That's why i moved it into loop's body. I'm also aware that null and false are not strictly equivalent. However, in respect to the scope of this exercise, that is besides the point.
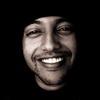
miikis
44,957 Points"That means it would be called every time, regardless of the loop."
No, it wouldn't. The loop only exits once secret equals "sesame." If secret doesn't equal "sesame," the loop runs forever — document.write would just never be called. I'm not saying that your way won't work, just that it's completely unnecessary... and a slippery slope, at that; why not wrap it all in separate functions and implement separation of concerns while you're at it? Katherine's problem was syntax ... and that's it. I didn't re-write the code for her because I thought she might find figuring it out the syntax herself to be more educational.
As a side note, you were wrong about correctGuess being null as well — it'd be undefined.
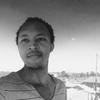
Dan Weru
47,649 PointsI ran it in the browser ... it runs both ways. You're right ... you're obviously seasoned in javascript than I'm. Pleasure.