Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial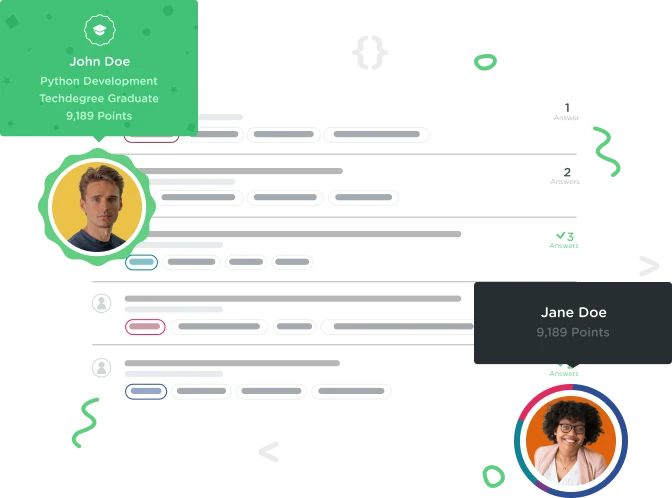

Robert Allred
920 PointsWhat am I missing?
What am I missing here? Because to me this looks like it should repeat this process of printing to the log the first number that is in the array, which also removes it and then repeats that process.
var temperatures = [100,90,99,80,70,65,30,10];
for ( var i = 0; i < temperatures.length; i += 1){
console.log(temperatures.shift());
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
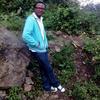
Charles Wanjohi
9,235 Pointsshift() method removes the first element in an array.Therefore every time the shift() method is executed, the length of the array is reduced: After executing the loop for 4 times, the length of the loop will be 4 because the first 4 elements will have been removed already.Therefore the (i < temperatures.length) condition in the for loop will not be satisfied i because the value for i is 4 by now .As a result the loop terminates . You can achieve the desired result by implementing the code as follows:
var temperatures = [100,90,99,80,70,65,30,10];
while ( temperatures.length>0){
console.log(temperatures.shift());
}
Hope this helps you
Nick Gentile
4,237 PointsNick Gentile
4,237 PointsI'm not sure what you are doing with .shift() -- but I can tell you that is where you are wrong.
What you want to do is log the value of temperature, at the given index point in the for loop (i).
Remember that to log a value at a certain index point of an array we do something like this:
array[index]
Big hint if you are still struggling ::
Your array is called TEMPERATURES
and the variable you are using to grab the index is [i]