Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial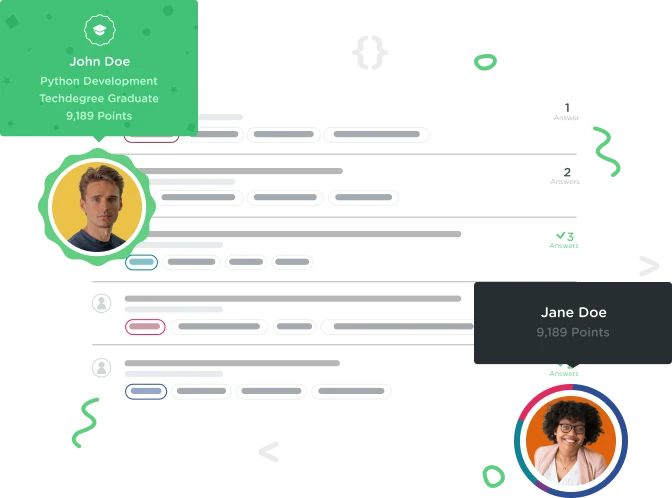
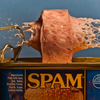
Scott Peake
17,052 PointsWhat am I missing?
I have watched the two preceding videos and I am still not able to get past this exercise. I was sure I knew what I was doing, but apparently not.
Asked to create a function that when it takes in an array, it returns the length of the array. When anything other than an array is passed in, it should return 0. Seems simple enough, but NO!
I created the function and used the name "ZIP" for the input (just because). Then I set an IF test, checking whether ZIP was an array...if it is, it returns the array's length. The "else" condition was to return a 0. Is that not what it's asking us to do?
Help!!
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter (zip) {
if (typeof zip === 'array') {
return zip.length;
}
return 0;
}
</script>
</body>
</html>
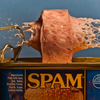
Scott Peake
17,052 PointsDuh! Object!
Thanks John! Not just a good answer, but a good WAY to answer!
1 Answer

Geoff Parsons
11,679 PointsUnfortunately javascript's typeof
doesn't return "array" as an option, you'll get "object" back when passing in an array. From the console:
var arr = [1,2,3]
typeof arr // -> returns "object"
You'll have to explicitly check for "undefined", "string", and "number":
function arrayCounter (zip) {
if (typeof zip === 'undefined' || typeof zip === 'string' || typeof zip === 'number') {
return 0;
}
return zip.length;
}
Checkout the MDN article on typeof for more info.
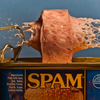
Scott Peake
17,052 PointsMy failure was the whole OBJECT vs. ARRAY. I was thinking I would need to do a more complicated "if" statement like the one you presented, but I wasn't sure how to do that (not familiar with the use of ||). I used the original "if" statement I included above, but simply adjusted the equality test to OBJECT from ARRAY. That passed the challenge.
John Magee
Courses Plus Student 9,058 PointsJohn Magee
Courses Plus Student 9,058 PointsThe typeof function might not return what you think it will return. Try it out in the console.