Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial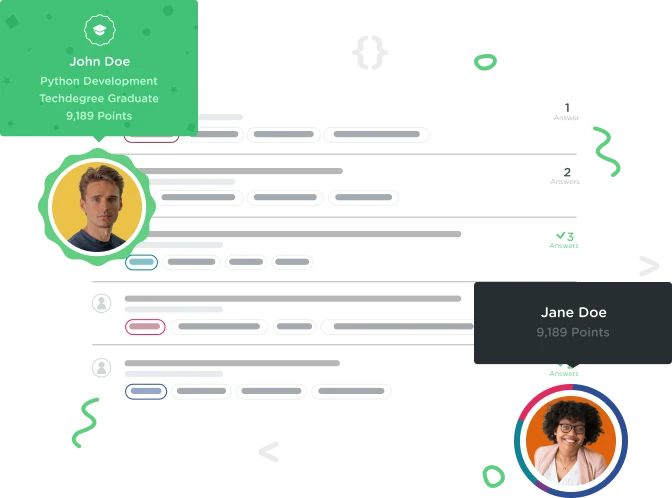

Keith Miller
2,310 PointsWhat am I missing here?
Any clues to what I am missing?
<?php
//edit this array
$name1 = array('name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com' );
$name2 = array('name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com');
$name3 = array('name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com');
$name4 = array('name' => 'Andrew Chalkey', 'email' => 'andrew.chalkley@teamtreehouse.com');
$contacts = array($name1, $name2, $name3, $name4);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>Alena Holligan : alena.holligan@teamtreehouse.com</li>\n";
echo "<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>\n";
echo "<li>Treasure Porth : treasure.porth@teamtreehouse.com</li>\n";
echo "<li>Andrew Chalkley : andrew.chalkley@teamtreehouse.com</li>\n";
echo "</ul>\n";
6 Answers

Keith Miller
2,310 PointsSo I figured out the spacing issue.
<?php
//edit this array
$contacts = array(array(
'name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com'), array(
'name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com'), array(
'name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com'),array(
'name' => 'Andrew Chalkley', 'email'=> 'andrew.chalkley@teamtreehouse.com', ));
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>". $contacts[0]['name'] . ' : ' . $contacts[0]['email'] ."</li>\n";
echo "<li>" . $contacts[1]['name'] . ' : ' . $contacts[1]['email'] ."</li>\n";
echo "<li>" . $contacts[2]['name'] . ' : ' . $contacts[2]['email'] ."</li>\n";
echo "<li>" . $contacts[3]['name'] . ' : ' . $contacts[3]['email'] ."</li>\n";
echo "</ul>\n";

Antonio De Rose
20,884 Points<?php
$name1 = array('name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com' );
$name2 = array('name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com');
$name3 = array('name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com');
$name4 = array('name' => 'Andrew Chalkey', 'email' => 'andrew.chalkley@teamtreehouse.com');
$contacts = array($name1, $name2, $name3, $name4);
#THIS WAY IT WILL SEND THE OUTPUT, AS REQUESTED, BUT THE WHOLE POINT HERE IS, TO
#STRUCTURE A MULTI DIMENSIONAL ARRAY, but your effort is worth, I'd say, only to say, you have only a minor fix
/*Think of only having the contacts variable and construct the same way, so one outer array, and bring in all your
arrays, make sure, you ignore all of your assignments like $name1 till $name4, you are going to only assign to
$contacts*/
$contacts = array(
xx,
xx,
xx,
xx
);
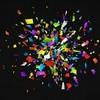
Synergi Tech
2,505 PointsHi, It's multidimensional. See the code below for reference
<?php
//edit this array
$contacts = array(array('name'=>'Alena Holligan'), array('name'=> 'Dave McFarland'), array('name'=>'Treasure Porth'),array('name'=> 'Andrew Chalkley'));
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>Alena Holligan : alena.holligan@teamtreehouse.com</li>\n";
echo "<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>\n";
echo "<li>Treasure Porth : treasure.porth@teamtreehouse.com</li>\n";
echo "<li>Andrew Chalkley : andrew.chalkley@teamtreehouse.com</li>\n";
echo "</ul>\n";
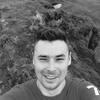
jamesjones21
9,260 Pointsyou can actually perform this by adding the array like so:
$contacts = [
//each multidimensional array
'contact1' => ['name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com' ],
'contact2' => ['name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com'],
'contact3' => ['name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com'],
'contact4' => ['name' => 'Andrew Chalkey', 'email' => 'andrew.chalkley@teamtreehouse.com']
];
Each <li> tag should follow the following format:
So we are calling the key then the associative key name.
echo "<li>" . $contacts['contact1']['name'] . ' : ' . $contacts['contact1']['email'] . "</li>\n";
echo "<li>" . $contacts['contact2']['name'] . ' : ' . $contacts['contact2']['email'] . "</li>\n";
echo "<li>" . $contacts['contact3']['name'] . ' : ' . $contacts['contact3']['email'] . "</li>\n";
echo "<li>" . $contacts['contact4']['name'] . ' : ' . $contacts['contact4']['email'] . "</li>\n";
for future lessons: you can loop through each of the arrays :)
foreach($contacts as $key => $value){
echo $value['name'] . ' ' . $value['email'] . '</br>';
}

Keith Miller
2,310 Points<?php
//edit this array
$contacts = array(array(
'name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com'), array(
'name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com'), array(
'name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com'),array(
'name' => 'Andrew Chalkley', 'email'=> 'andrew.chalkley@teamtreehouse.com', ));
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>". $contacts[0]['name'] . ' : ' . $contacts[0]['email'] ."
</li>\n";
echo "<li>" . $contacts[1]['name'] . ' : ' . $contacts[1]['email'] ."
</li>\n";
echo "<li>" . $contacts[2]['name'] . ' : ' . $contacts[2]['email'] ."
</li>\n";
echo "<li>" . $contacts[3]['name'] . ' : ' . $contacts[3]['email'] ."
</li>\n";
echo "</ul>\n";
Thanks for the help guys! I used a combination of both James and Harry's suggestions. Now I am getting what seems to be the correct output but am being told to check my spacing. I've gone through a number of spacing options. I am assuming the spacing issue is around the colon...

Michel Ribbens
18,417 Pointscan somebody explain why we need to include the first and last dots
don't understand why including the code $contacts[0]['name'] . ' : ' . $contacts[0]['email'] isn't enough and why we have to include the dots after and before the li tags
"<li>".
."<li>"
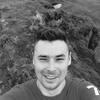
jamesjones21
9,260 Pointsas we are concatenating a string, the full stops will add this into one string:
<li>Dave McFarland : dave.mcfarland@teamtreehouse.com</li>
the contact name will then be pulled into the $contact[0]['name'] placeholder and then $contact[0]['email'] will be pulled into the email placeholder. Also because this is a test, when checking the answers it will take in account the spacing and the html, hence the last dots.
hope this helps.
Kind regards, James
jamesjones21
9,260 Pointsjamesjones21
9,260 Pointswith a lot of concatenation, and the double quotes it can get a lot out of hand unfortunately, one thing that Aleana doesn't teach here is the short hand methods when it comes to outputting html in the loops , which I find short hand much better for outputting things to the screen.
but for echo's you may use:
it will become much clearer when you look at looping through the arrays to output to the screen at later stages in the course, if you do get stuck send me an email: jamesjonestred@gmail.com I'll hopefully be on hand to help.