Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial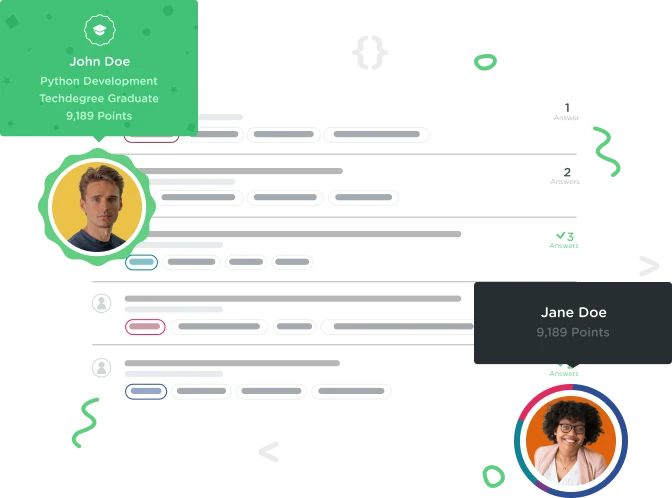
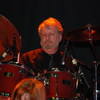
Ralph Johnson
10,408 PointsWhat am I missing here?
"Bummer! Remember, belongs_to
always wants the singular version of the relationship."
(most frequent "hint" message is that "has_many" goes with "BlogPost" and "belongs_to" goes with "Comments")
`1 class BlogPost < ActiveRecord::Base
2 has_many :comments
3 endβ
4
5 class Comment < ActiveRecord::Base
6 belongs_to :blogpost
7 end`
I don't get it. I've tried this with CamelCase, tried switching the relationships (which makes no sense but I figured "what the hell"), and no matter what combination (sometimes more than one statement under the classes) everything gets kicked out.
4 Answers
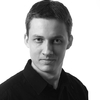
Maciej Czuchnowski
36,441 Pointsclass BlogPost < ActiveRecord::Base has_many :Comments end
comments
should be lower case. It's all case sensitive. the code that passes this exercise:
class BlogPost < ActiveRecord::Base
has_many :comments
end
class Comment < ActiveRecord::Base
belongs_to :blog_post
end
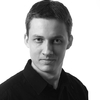
Maciej Czuchnowski
36,441 Pointsbelongs_to :blog_post
Look at the exercise. It says blog_post_id
, which means the blogpost should actually be blog_post
. The name of the model BlogPost tells you the same thing - the capitalization suggests underscores.
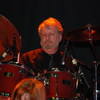
Ralph Johnson
10,408 PointsTried that.
Bummer! Has_many
should be on BlogPost
.
Then:
`class BlogPost < ActiveRecord::Base has_many :Comments end
class Comment < ActiveRecord::Base belongs_to :blog_post end`
doesn't seem to work either. Also tried it without the colon, ( belongs_to blog_post
) and got:
Bummer! NameError: undefined local variable or method `blog_post' for Comment(Table doesn't exist):Class
Then I tried this:
class Comment < ActiveRecord::Base
belongs_to :BlogPost.blog_post_id
end
Bummer! NoMethodError: undefined method `blog_post_id' for :BlogPost:Symbol
Why (based on the first "Bummer!" statement) would a Comment have_many BlogPosts? That doesn't make any sense.
What does Has_many
should be "on" BlogPost
even mean?
Even tried this (which makes no sense):
`class BlogPost < ActiveRecord::Base belongs_to :Comment end
class Comment < ActiveRecord::Base has_many :BlogPosts
end`
and got this:
Bummer! Remember, has_many
should be on BlogPost
and belongs_to
should be on Comment
.
Still don't understand how blog_post_id factors into all this.
I checked the ActiveRecord reference here: http://api.rubyonrails.org/classes/ActiveRecord/Associations/ClassMethods.html and it looks like I'm formatting my statements correctly (at least in terms of using :symbols and not multi_underscore_variables).
There is obviously something ELSE I'm missing. I've tried all sorts of statements and formatting, and still keep getting an error.

Jonathan Carrroll
746 Pointshey i just want to know how to center my letters so if you know please respond
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsI know it's all very confusing, but you will start to naturally see patterns in all this (i.e. when to use camel case, when to use plurals, when to use symbol notation, when to use underscores etc.). Been there. The only thing I can tell you is to be strong, don't give up, persevere :)
Ralph Johnson
10,408 PointsRalph Johnson
10,408 PointsThank you, Maciej, for YOUR persistence in answering questions over long exchanges. I haven't given up yet, and probably won't if I don't die first :).
The official Ruby reference showed no multi-word symbols--that I could see, anyway, so I couldn't see any underscored examples--that might have given me a clue.
But it also showed no upper case letters in the symbols, even though the associated class names were capitalized, so I should have gotten that part.
Solving the exercise just involved "too many variables," if you'll pardon the pun, so a/b testing was sorta tough.
And I'm still getting messed up by instance variables (@var), local variables(var) and so on, but that's because in rails all the code is broken up between dozens of files--so, for instance, a variable defined inside a method in one file is used (with no embellishment) in a completely different file, and it gets hard to keep straight--in these lessons I keep asking, "where did THAT variable come from--did he pull it out of his @#$?"
The teachers are very fast at this stuff, and sometimes they'll finish typing something, save the file, and be off to the next screen before I've digested 10% of what they're talking about--and if I'm following along, I often have to pause and rewind just to READ the code, let alone copy it.
The ultra-basic courses seem to go too slow (I suppose that's because I've already taken almost all the courses in the codecademy library, covering a lot of this stuff) but the more intermediate ones in Treehouse fly at just below the speed of sound, with a lot of stuff glossed by with little or no explanation.
Rails may be "of" Ruby, and written "in" Ruby, but Ruby and rails are not the same thing at ALL when it comes to teaching/learning--when the code resides in 12 different files and refers to things (like class definitions) that may or may NOT be referred to in the video window, and whose cause-effect relationship is never fully explained, AND we're jumping back and forth between irb, the terminal console, the rails console, and 3 different files that all deal with the same table information...well, getting my capitilization-underscoring-CamelCase right (and remembering where each of those things comes from) is a bit overwhelming.
That effect is heightened even more when a temporary variable used between the pipes in an iteration is named the same as something more permanent--I wish they wouldn't do that. That's why I try not to make the temp variable names too similar--so I can keep it straight in my head as I get 7 or 8 lines down the page and also using instance or class variables (from somewhere else) within the same method--though I can see why in a body of code you're handing to somebody else it might not be such a good idea to name your temp variable 'x,' or "beef" when you're dealing with a program about iron ore, it sure as hell helps me to keep things straight.
When I was first learning Ruby, and a variable (like a local or instance variable) was described as only working "within a class," I could SEE where the class definition started and ended, and where the "action" code started and ended. When a single interactive concept is spread across 6 different files, how the heck do you tell if you're "in" the class or outside it?
It's hard enough INSIDE a single-page program to see cause and effect--you're trying to figure out whether the error message you just got was because you threw a fastball or a curve ball, only to find out it's because the center fielder was (or wasn't) mooning the bullpen at the time.
I think that even though DRY is the paradigm for writing code, it's probably not a good idea for teaching a long course broken up over a hundred or so videos--especially when the settings (which file you're in, etc) keep constantly changing and getting more complicated. Even when you've learned something and have it halfway mastered, seeing the same thing in a completely new context can throw you pretty hard. The teachers probably need to coordinate and compare notes with each other better, too.
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsI can only suggest looking into other sources as well to see stuff from different perspectives. At some point it will simply click. I had to start and play around with like 5 or 6 projects before I got most of the things (yeah, I'm slow, but thorough). You could try this, it's free and uses the same version of Rails as Odot (the newer version of the book has a lot of stuff changed):
http://rails-4-0.railstutorial.org/book
Maybe this will help you somehow.