Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial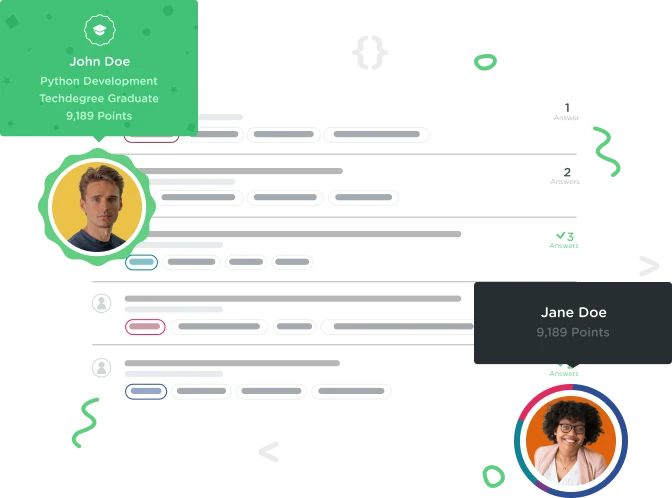

arshia mohammadi
7,657 PointsWhat am I missing in this code challenge??
Create a new function named max which accepts two numbers as arguments (you can name the arguments, whatever you would like). The function should return the larger of the two numbers. HINT: You'll need to use a conditional statement to test the 2 parameters to see which is the larger of the two.
thanks for your help!
function max (number1, number2) {
if (number1 > number2) {
return number1;
return number2;
}
max (60,4);
}
2 Answers

Ross King
20,704 PointsHi Arshia,
The comment by Adam Beer is correct. The function will return the max number.
function max(num1, num2) {
if (num1 > num2) {
return num1;
}
return num2;
}
Also, you had include your function call inside the function. ensure you add max (60,4); outside the function call.
As victor pointed out in comments, this function will return num2 even if num1 are the same, however, if you put pass the numbers (20,20), then num2 will return 20 which is the highest number and technically correct.
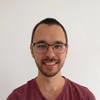
victor cooper
6,436 PointsA return will will give back the value and will exit the function, so if number1 is bigger than number2 you will return number1, the code execution will never reach return number2.
But that's not the problem. You only check if number1 is bigger than number2, you need to also check if number2 is bigger than number1.
if ( number1 > number2) {
return number1;
} else if ( number1 < number2 ) {
return number2;
}
Hope this helps.
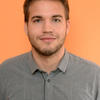
Adam Beer
11,314 PointsI know a third way, this is the shorthand way :)
function max(num1, num2) {
if (num1 > num2) {
return num1;
}
return num2;
}
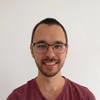
victor cooper
6,436 PointsThis function will return num2 even if both are the same, not only if num2 is bigger than num1
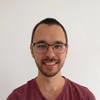
victor cooper
6,436 PointsWhoops, i had the same mistake with my first option. Removed it. Thanks to your comment i noticed it =]
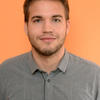
Adam Beer
11,314 PointsInteresting. I tried it now and returned the larger number :)
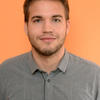
Adam Beer
11,314 PointsHave a nice day! :)

Ross King
20,704 PointsHi Victor,
The conditional will return undefined if number1 and number2 and not the max number. If both numbers passed to function are the same it would not matter which are returned as they are both the highest.
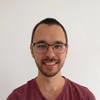
victor cooper
6,436 PointsMy function only checks to see if one number is bigger than the other. If you will pass two of the same numbers ( lets say 12 and 12 ), than both checks will fail and the function will end without any output.
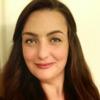
Jennifer Nordell
Treehouse TeacherAdam Beer Since you mentioned brevity, I thought I would throw out an answer that is even shorter. Take a look
function max(x, y) {
return x > y ? x : y;
}
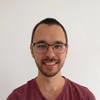
victor cooper
6,436 PointsA nice and short check bu still it will give you an answer if both the numbers are the same. Again 10 is not larger than 10 and so if the function checks which numer is larger you will get a wrong answer.
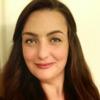
Jennifer Nordell
Treehouse Teachervictor saltanov No, that would be correct. Of the data set 10 and 10, 10 is the maximum value. There is no value in that data set that is above 10 The only way it should not return a value is if the data set was empty to start with.

Ross King
20,704 PointsJennifer Nordell throwing in Ternary operators is just mean :) Should of minified it as well!
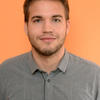
Adam Beer
11,314 PointsHahahah, I forget the ternary operator. Well done, Jennifer, you are right, again!
victor cooper
6,436 Pointsvictor cooper
6,436 PointsI have to disagree. If you must check if one option is larger than the other than this function is not correct. 20 is not larger than 20, and if the function will return an answer of 20 then it is the wrong answer.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse Teachervictor saltanov I disagree. Let's not say it's 2 numbers, let's say it's 4 and we want the max number. Let's go one step further and say it's ages. We have 4 people and the following ages, [21, 20, 44, 44]. By your logic, we would exclude the two 44 year-olds and say that the max age is 21 and that isn't correct. The
You can find the MDN documentation here.
max
value of something should be just that, the maximum of the data set, regardless of what is in the data set. To be clear, this is how theMath.max()
included in JavaScript works as well. TryMath.max(20, 20);
in your consoleRoss King
20,704 PointsRoss King
20,704 PointsDisregard my deleted comment, Jenn beat me to it! Thanks for the comment.
victor cooper
6,436 Pointsvictor cooper
6,436 PointsHey when you are wrong you must learn from it, and i was wrong. Thanks for that example, I dont think i would have changed my mind about my function if you would not gave me that example. Thank you jennifer, that was something my stubborn head needed XD