Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial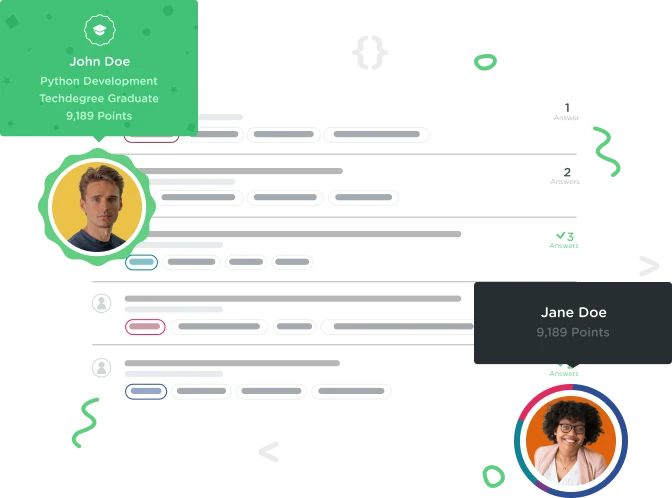
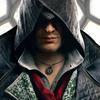
Gerard Nwazk
Courses Plus Student 2,833 PointsWhat am i not doing right here? pls correct me!...
Challenge Task 1 of 1
Alright, here's a fun task! Create a function named combiner that takes a single argument, which will be a list made up of strings and numbers. Return a single string that is a combination of all of the strings in the list and then the sum of all of the numbers. For example, with the input ["apple", 5.2, "dog", 8], combiner would return "appledog13.2". Be sure to use isinstance to solve this as I might try to trick you.
Am i doing it right or am i missing somthing?
# input argument
# list to contain arguments
# loop through the list
# if the list isinstance(str)
# if the list isinstance(int)
# return arg_list
def combiner(args):
arg_list = []
string_arg = []
string_arg.append(args)
int_arg = []
int_arg.append(args)
if arg_list:
for arg_str in arg_list:
if isinstance(arg_str, str):
return string_arg.append(arg_str)
for arg_int in arg_list:
if isinstance(arg_int, int):
return int_arg.append(arg_int)
return arg_list.extend(string_arg, int_arg)
3 Answers
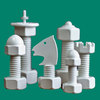
Steven Parker
229,744 PointsHere's a few hints:
- you may not want to append the entire "args" list to both new sub-lists
- you might want to put something into arg_list before testing it, an empty list is always "falsey"
- you might want to check all the items in the list before returning
- you're testing for int and str values, but what about float?
- you still need to combine numbers by adding them

Unsubscribed User
3,284 PointsYou don't really need to create two new lists and do if arg_list. Instead, it might be easier to tackle this problem if you turn the input into a list or just except the input's gonna be a list, since you and I already know it's gonna be a list. Then just loop through the list, and check if it's a string or an integer or a float. maybe you could define a global variable to concatenate the strings and increment the numbers, and then just combine the two in the end.
However, you can still go with this approach though. To me, it makes sense if you, in the beginning, append args into your new list and check if that list has any item inside it. and loop through that list and have two if statements instead of two for loops.

Leroy Saldanha
8,006 Pointsdef combiner(single): give_back=[] add_numbers = 0 for x in single: if isinstance(x,(int,float)): add_numbers += x if isinstance(x,(str)): give_back.append(x) give_back.append(str(add_numbers)) return ''.join(give_back)