Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial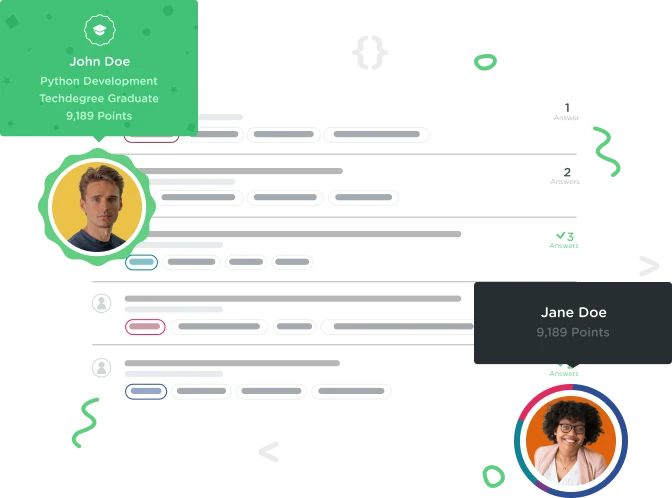

Tojo Alex
Courses Plus Student 13,331 PointsWhat am I suppose to do
I really need help, help very much appreciated.
<?xml version="1.0" encoding="utf-8"?>
<manifest package="com.teamtreehouse.timetraveler"
xmlns:android="http://schemas.android.com/apk/res/android">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme"
reciever android:name="com.teamtreehouse.intent.action.RIFT"
>
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
<activity android:name=".TimeTravelActivity">
<intent-filter>
<action android:name="android.intent.action.SEND"/>
<category android:name="android.intent.category.DEFAULT"/>
<data android:mimeType="text/plain"/>
<data android:mimeType="image/*"/>
<data android:mimeType="video/*"/>
</intent-filter>
</activity>
</application>
</manifest>
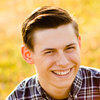
Caleb Kleveter
Treehouse Moderator 37,862 PointsWhat is the problem you have? You should explain that in your question so people know what to look for to help fix the issue.
5 Answers

Seth Kroger
56,413 PointsWhat you need to do is add a <receiver>
tag inside the <application>
:
<?xml version="1.0" encoding="utf-8"?>
<manifest package="com.teamtreehouse.timetraveler"
xmlns:android="http://schemas.android.com/apk/res/android">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
<receiver android:name="com.teamtreehouse.intent.action.RIFT" />
</intent-filter>
</activity>
<activity android:name=".TimeTravelActivity">
<intent-filter>
<action android:name="android.intent.action.SEND"/>
<category android:name="android.intent.category.DEFAULT"/>
<data android:mimeType="text/plain"/>
<data android:mimeType="image/*"/>
<data android:mimeType="video/*"/>
</intent-filter>
</activity>
<receiver android:name="...">
<!-- Other tags like intent-filter for the receiver go here -->
</receiver>
</application>
</manifest>

Gift Nyamapfene
5,409 Points<?xml version="1.0" encoding="utf-8"?> <manifest package="com.teamtreehouse.timetraveler" xmlns:android="http://schemas.android.com/apk/res/android">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
<receiver android:name="com.teamtreehouse.intent.action.RIFT" />
</intent-filter>
</activity>
<activity android:name=".TimeTravelActivity">
<intent-filter>
<action android:name="android.intent.action.SEND"/>
<category android:name="android.intent.category.DEFAULT"/>
<data android:mimeType="text/plain"/>
<data android:mimeType="image/*"/>
<data android:mimeType="video/*"/>
</intent-filter>
</activity>
<receiver android:name=".RiftReceiver">
<intent-filter>
<action android:name="com.teamtreehouse.intent.action.RIFT"/>
</intent-filter>
</receiver>
</application>
</manifest>

Tojo Alex
Courses Plus Student 13,331 PointsQUESTION : Now we want to update our time travel app to monitor for broadcasts about rifts in the space-time continuum. Add a manifest receiver that listens for these broadcast events. For the receiver class, use "RiftReceiver", and for the action, use "com.teamtreehouse.intent.action.RIFT".
ERROR (not in compiler) : Bummer: Looks like you didn't add a receiver inside the application element.
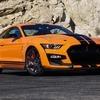
Munashe Shava
6,789 Pointshi
Tojo Alex
Courses Plus Student 13,331 PointsTojo Alex
Courses Plus Student 13,331 PointsUpdate :