Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial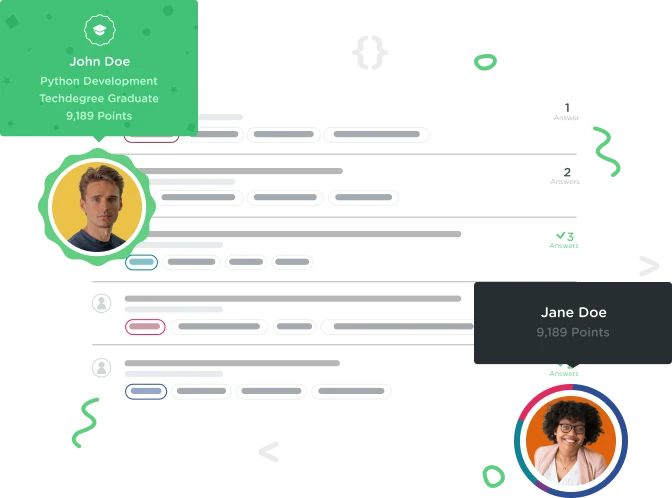
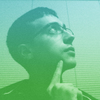
Luke Riley
10,403 PointsWhat am I supposed to do
I'm not quite sure what the question is asking me to do exactly. The question is kind of vague.
3 Answers
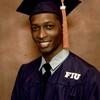
Dane Parchment
Treehouse Moderator 11,075 PointsAlright let me walk you through this answer, before I begin, I want you to stop and think for a moment ask yourself these questions: What is this question asking me to do? How can I go about creating a solution for this question? What do I know?
What is the question asking me to do?
- Create a function called arrayCounter
- Function must take one parameter that is an array
- Return the length of the array
- If the parameter is a number, string, or is undefined Return 0
What do I know? For this type of challenge I believe you should:
- Be comfortable writing variables
- Be comfortable using if statements/else if statements
- How to write a function, and what parameters are
- Basic JavaScript syntax
How can I go about creating a solution for this question? If you haven't already guessed in the first question that I answered the italicized words should give hints onto what we will be using to solve this type of challenge. Now get ready for the walkthrough.
First we need to create a function called arrayCounter, here we will make the basic skeleton of that function.
function arrayCounter() {
}
Next we need to add a parameter to this function, I will call it 'array' but you can call it whatever you like.
function arrayCounter(array) {
}
We are required to return the length of the array, and as such we know to use the length statement. So let's create that return statement.
function arrayCounter(array) {
return array.length;
}
Now that is all fine and dandy, but now we need to check for the type of parameter the function is using. We should know of a statement called typeof by now that will return the type of the variable that it is affecting. Now there are multiple ways we could go about doing this, but I find that using a variable to store the type of the parameter and then comparing it in if statements is most affective.
function arrayCounter(array) {
var paramType = typeof array;
if(paramType === "undefined") { //are you undefined
return 0;
}
else if(paramType === "number") {//are you a number
return 0;
}
else if(paramType === "string") {//are you a string
return 0;
}
return array.length; //must be an array!
}
Now for readabilities sake I used 1 if statement and two else ifs but you could have easily used one if statement like so.
function arrayCounter(array) {
var paramType = typeof array;
if(paramType === "undefined" || paramType === "number" || paramType === "string") { //are you undefined, a number, or a string
return 0;
}
return array.length; //must be an array!
}
Hopefully this was of assistance to you! I recommend that you always ask those questions and do your best of answering them whenever you come across another hard challenge, or a real-world programming problem, I find that it helps make you think like a programmer! Good luck and have fun!

Ben Falk
3,167 PointsQuestion: "Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in."
- it is asking you to create a function named "arrayCounter"
- The function needs to accept one parameter, which is an array
- The function checks that the item passed into the function is an array. If it is an array, the function returns the length of the array (probably using ".length"). If the item passed into the function is not an array, then return 0.
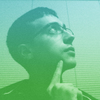
Luke Riley
10,403 PointsThanks! This was helpful! I should have posted my code. This is my first question on the forums.
I did not originally understand about the numbers and string part. The only thing I understood that it wanted me to create the function and name a parameter see if it was undefined and, if not print its length.
My original code was this (missing the number and string) '''html <script> function arrayCounter(array) { if(typeof array === "undefined") { return 0; } return array.length; } </script> ''' Also, thanks for showing me how to simplify it using the variable and combining the if statements!
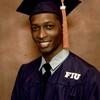
Dane Parchment
Treehouse Moderator 11,075 PointsNo problem glad to be of assistance!