Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial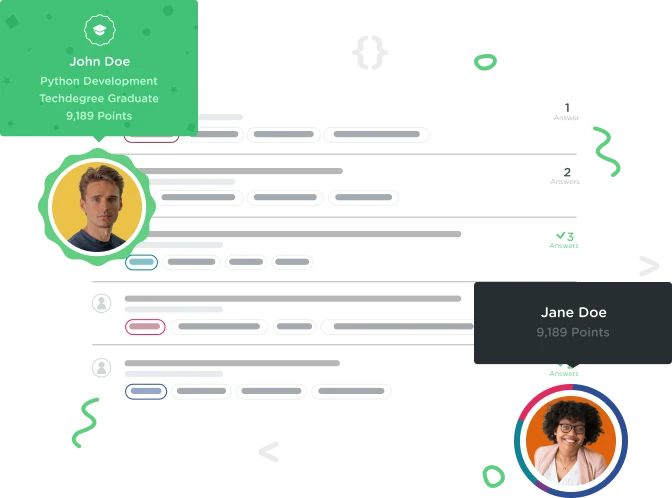

Conner Wells
5,979 PointsWhat am I supposed to put in the do loop in order for this to work?
I thought I could just state the "secret" variable in order for the variable to be added, but I keep getting the error that the variable has to be defined outside of the loop, but I'm also getting an error that says that the prompt() function must be used inside the loop. I'm really lost and confused.
var secret = prompt("What is the secret password?");
do {
secret;
}
while ( secret !== "sesame" ) {
document.write("You know the secret password. Welcome.");
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
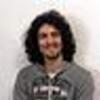
Gergely Bocz
14,244 PointsHi Conner!
First here is a quick summary of the do-while loop: The do-while loop first executes the code inside do, no matter what. Then checks the condition inside while, and based on the condition's return value, it can do 2 things. If the condition is true, then we execute the code inside do again. If the condition is false, then we leave the do-while loop. Essentially it is a while loop that executes at least once.
Please check out the syntax for do-while loops either in the video or here for example, because you didn't write a do-while loop, you wrote something like a do loop - that doesn't exist! - followed by a while loop.
So all we want to do is rewrite our while loop into a do-while loop. Since the code inside do gets called no matter what, we want to write something inside, that can get called, no matter what. This is the reason for your errors. If you define a variable (secret) with a function call to it (prompt), then that function will be called immediately! In short, prompt gets called before we enter the loop, and there is no need for that, just declare the variable without any assignment. But we want to keep on calling prompt as long as the given password is incorrect, so we put it inside the loop. Basically we want to declare the secret variable without assignment because of the do part of the loop, and we want to call prompt every time, the password is not sesame, so we put that inside the loop. Hope it makes sense!
If you want to see how your code behaves with
var secret = prompt("What is the secret password?");
just open the dev tools console and paste your code there and try writing the correct password, "sesame" in there, and see for yourself, that it won't work the first time, you'll have to write it again!
Try to rewrite your code and find the solution yourself, if you need further help just ask for it, and i'll try to help the best i can!
Justyna Szofinska
6,178 PointsJustyna Szofinska
6,178 PointsI did it this way. It seems to me, that your code doesn't work, because you didn't clearly say what the program should do when the answer is correct or uncorrect.