Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial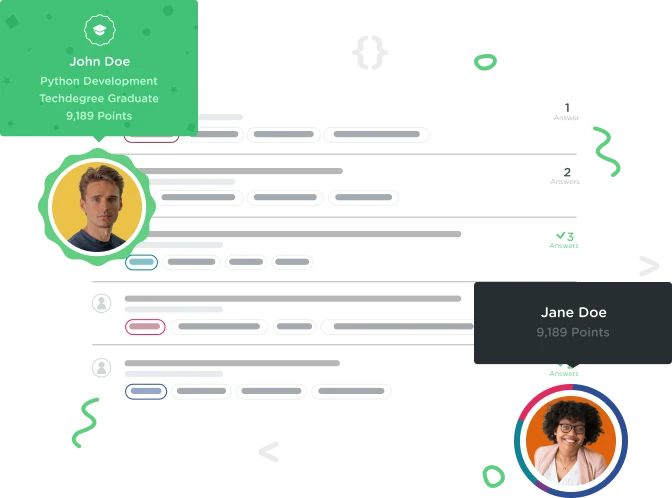

babasariffodeen
6,475 PointsWhat are callbacks? And how does the 'this' keyword work in a function?
LINK TO VIDEO: https://teamtreehouse.com/library/basic-arrow-syntax
In this video it is mentioned that arrow functions are beneficial to callbacks, but what is a callback?
Also, in the teachers notes, the following code is given: unction Person() { this.age = 0;
var self = this;
setInterval(function() { self.age ++; }, 1000);
}
However, I don't understand how 'this' is being used here. There hasn't been an explanation of 'this' so I would love to get some explanation about it, and the code explained please?
2 Answers

Victoria Retallick
11,127 PointsA callback is a function of code that can be run later, usually passed in as a parameter of another function. The callback can be a named function (defined elsewhere) or an anonymous function (inline function() {}) For example, the native JS function setTimeout here would open an alert after 5 seconds:
setTimeout(function(){ alert('Hello!'); }, 5000);
In vanilla javascript the 'this' reference can change depending on the scope of the code. Using arrow functions now we get around this and 'this' remains static. It is a nice change and gets around multiple uses of that = this or self = this :) But basically you use this to change the variables of that scope. If you defined age = 0; you would be changing the global scope and that might clash with some other variable name. However if you just used a var it might be difficult to locate in scope, especially with lots of nested code. So usually variables are added to the top of a js file and wrapped in a function.
See here for more on this: https://javascriptplayground.com/javascript-variable-scope-this/
If you have any more questions, let me know. I might not have explained it very well.

Alexander La Bianca
15,959 PointsA callback is a function that is provided as an argument to another function. Consider a simple click handler
button.onClick(function() {
//this is the callback function
});
button.onClick(callbackfunction)
function callbackfunction() {
//this is the same as the callback functon above
}
It was always hard to wrap my head around the fact that we can put functions as arguments into javascript functions. Until I started writing my own functions that accept callbacks. Consider this example:
function aFunctionThatAcceptsACallback(value1,value2,callback) {
setTimeout(function(){ //setTimeout just to fake something async
const sum = value1 + value2;
callback(sum);
},1000);
}
//now we can use this
aFunctionThatAcceptACallback(1,2,function(sum) {
console.log(sum) //will log out 3 after one second
});
Functions usually accept callbacks if it involves some async action