Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial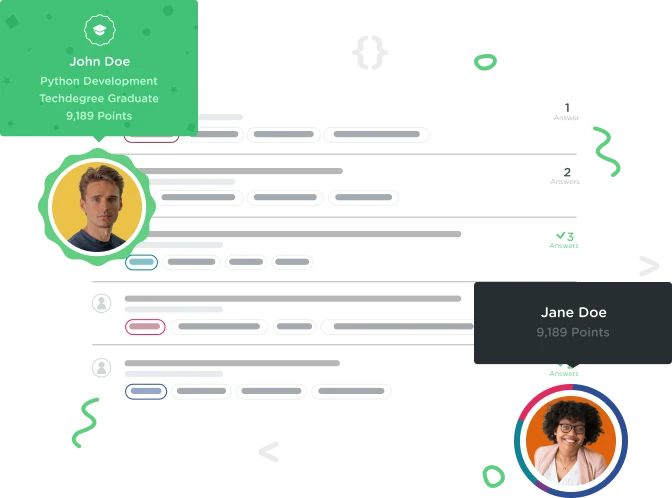

wwyattw
10,389 PointsWhat are the differences between if and switch operator?
What are the differences between if and switch operator? What are the advantages and disadvantages?
1 Answer
Brenden Konnagan
16,858 PointsThey are both control flow statements.
Perhaps this will help:
let testValue = true
You will notice that testValue is a Bool assigned to true.
But what if you want to build logic on that? Traditional if / else statements might look like this:
if testValue {
// Do something for testValue is true
} else {
// Do something for testValue is false
}
Where a switch statement can be written like so:
switch testValue {
case true:
// Do something
case false:
// Do something
}
Really it is a matter of style, and logic. If you are testing for one condition, then a simple if / else statement might work. Otherwise, switch statements give you power to concisely and clearly express your intentions for a number of scenarios. This becomes very evident in a long if / else statement like:
if someValue {
// Do something
} else if someOtherCondition {
// Do something
} else {
// Do another thing
}
There are of course more advanced things you can do with switch statements as well, and coupled with Enums are quite powerful. I would recommend checking out the Apple Swift E-Book under the chapter on control statements for a further look at the switch statement.
Hope this helps!
Cheers!
wwyattw
10,389 Pointswwyattw
10,389 PointsI didn't notice your answer until now. Thank you so much this was very helpful. it's confusing sometimes because there are so many similarities.