Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial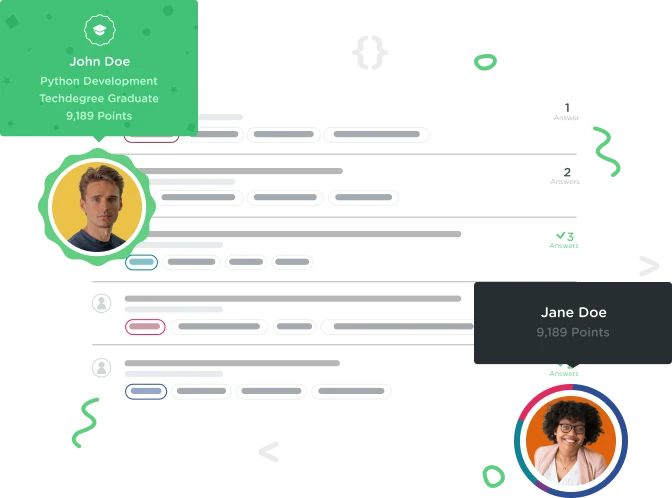
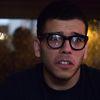
Luis Paulino
Courses Plus Student 1,779 PointsWhat are the internationalizes, conditions, and instants that I need to fill out?
If, I wrote this wrong please forgive me. I'm getting better and wiser each day, sooo I would just like a clue on what I need to do? Thank You for your help!
NSArray *drinks=@[@"juice",@"water",@"coffee"];
for( initializion; condition; incendent){}
3 Answers
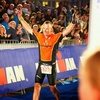
Steve Hunter
57,712 PointsHi Luis
You are talking about a long-hand for
loop where an integer is created for the loop counter, tested against a condition to see if the loop should continue, then amended to create a changing iteration. That's not quite what the challenge is looking for here.
For this, you can use the for in
syntax. You still need to create a new variable but the increment and condition are handled behind the scenes.
The array you are accessing called drinks
is an array of string literals. You want to extract each string in
the array so you need to be able to handle a string data-type. The syntax is for(NSString drink in drinks){}
where drink
is a local variable which could be called anything; for(NSString tipple in drinks){}
is just as correct, if terribly English.
Once you have extracted each drink
from the drinks
array, use an NSLog
to output that string to the log. The loop will iterate over each entry in the drinks
rray, pulling out each element one-at-a-time and storing that in the local variable drink
for the duration of that loop iteration:
NSArray *drinks = @[@"juice", @"water", @"coffee"];
for(NSString *drink in drinks){
NSLog(@"%@", drink);
}
I hope that helps.
Steve.
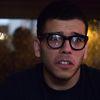
Luis Paulino
Courses Plus Student 1,779 PointsBut, How would you think "What do I put in this for in loop?" so, I don't get confused later on into the future.
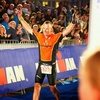
Steve Hunter
57,712 PointsTo decide whether to use a for
loop, you should understand first if there is a set number of loops required. So, if you're iterating through an array, a for
loop is perfect. If you're waiting for a user to input a specific word, a for
loop isn't for you! Use a while
loop, or something similar.
There are two constructs of a for loop. The first, the long-hand mthod, is what you initially asked about. It takes a counter, a condition upon that counter to stop the loop, and something that changes the counter so we don't run forever. In Objective C it would look something like:
int a;
for( a = 0; a < 20; a = a + 1 )
{
NSLog(@"value of a: %d\n", a);
}
(You may be able to declare the int
inside the loop like this: for(int a = 0, a < 20, a = a + 1)
. You can also manage the increment more easily by using a++
rather than the long-winded a = a + 1
)
The short-hand version, removes the need for the condition and the increment. The example above is using integers to demonstate the loop, this isn't ideal as to show the shorthand version, the for in
loop, needs a range of numbers which may confuse you. Take [@0 to:@19]
- this is a range of numbers from zero to nineteen - just like in the example above. Rather than coding the counter to start at zero and stop at not-less-than-twenty, we can use a range of 0 to 19 just the same. (There is one difference that we can cover later!). So, the for in
loop would take this range and rather than us having to tell the loop where to start and end, it just does it for us. We do need something to hold each iteration, though, so a variable of the appropriate data type is still required:
for(NSNumber *num in [@0 to:@19]){
// do something with num
}
So, if you have a collection of objects; arrays, dictionaries, JSON files, sets or other such things - iterating through these is best done with a for
loop. Using the short-hand syntax is easier than the longhand, but you can do it the long way if you want. Let's say your array NSArray *array;
has a method called count
that returns the number of elements in the array - it does, I think. You could than do something like:
NSArray *array = [// create an array in here];
int i; // maybe do this inside the loop
for(i = 0; i < array.count; i++){
// do something in here:
Log(@"%@", array[i]);
}
That was a rubbish example; sorry!
The one difference that long-hand can make is the ability to alter the route of execution. This is really bad practice so don't do it, but rather than just incrementing i
you can mess with its value if you want. Say, you want to start looping again if one of the array elements contained "Steve". There's no reason why you would want to do this - but go with it for a moment ...
NSArray *array = [// create an array in here];
int i; // maybe do this inside the loop
for(i = 0; i < array.count; i++){
// do something in here:
Log(@"%@", array[i]);
if(array[i] == @"Steve"){
i = 0; // starts the loop again!
}
}
Here, we've messed with the value of i
and set it to zero. This will restart the loop. We could have set it to anything! Worse, we could have set i
to greater than array.count
` which would cause all sorts of problems! So, don't mess with your loop counter - it's a good rule but I thought I'd show you to demonstrate the point.
Anything else I can cover for you?
Steve.
P.S. My Objective-C is very rusty so please accept my apologies for any bad code syntax!
Luis Paulino
Courses Plus Student 1,779 PointsLuis Paulino
Courses Plus Student 1,779 Pointsit really does help...thanks Steve. I still have a lot to learn, but I'm glad you help me. I'm really great-full to you.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem!