Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial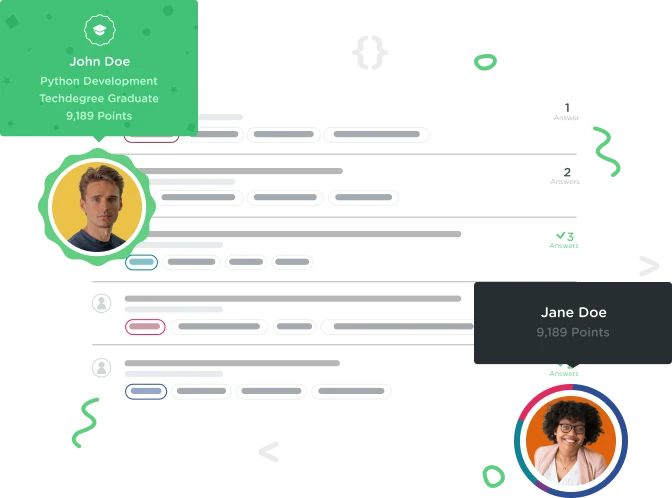

Alexandra Kaplan
1,167 PointsWhat are you doing to a code when you write index++?
In the code:
numbers = ["1","2","3"]
var index = 0
while index < numbers.count {println (numbers[index])
index++
}
What is the purpose of the index++ ? My code does not work until I add that.
2 Answers
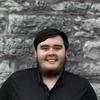
Michael Hulet
47,912 PointsWriting "++
" after a number variable adds 1 to that number. This is called "incrementing" the variable. In this case, index
is part of the condition of the while
loop. The loop will run as long as index
is less than the count
of the numbers
array. If you never increment index
, the loop will run forever (this is called an "infinite loop"), and will crash your app. Here's line-by-line what your code does:
let numbers = ["1","2","3"] //Declares an array constant of ["1", "2", "3] and sets it to numbers
var index = 0 //Declares an Int variable of 0 and sets it to index
while index < numbers.count { //Runs the code in the block as long as index is less than the number of items in the numbers array
println (numbers[index]) //Prints the number at the index of the numbers array at the current value of the index variable to the console
index++ //Increments, or adds one, to the index variable
}

akshaykhullar
6,943 Pointsindex++ increments the variable index by 1. Initially the variable index is set to 0. When the loop runs for the first time, the output is 1 ( numbers[0] ). The next line adds 1 to the index therefore the next time the loop runs it will output 2 ( numbers[1] ). Hope this helps!

Alexandra Kaplan
1,167 PointsAwesome, much appreciated
Alexandra Kaplan
1,167 PointsAlexandra Kaplan
1,167 PointsCan you explain why "if you never increment index, the loop will run forever"? This core concept I don't understand-what is making the loop run forever?
Michael Hulet
47,912 PointsMichael Hulet
47,912 PointsEvery time the code in the loop runs, the loop checks the value of
index
and compares it to the value ofnumbers.count
(which represents the number of items in thenumbers
array). Ifindex
is less thannumbers.count
, then the code in the loop runs. Ifindex
is greater than or equal tonumbers.count
, then the code in the loop is skipped, and your code moves on to whatever comes after thatAlexandra Kaplan
1,167 PointsAlexandra Kaplan
1,167 PointsI think I understand! So because the index number is equal to 0, the count of the numbers in the array will always be greater, so the statement will always be true and it will run forever?
Michael Hulet
47,912 PointsMichael Hulet
47,912 PointsExactly!
Alexandra Kaplan
1,167 PointsAlexandra Kaplan
1,167 PointsLike :)