Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial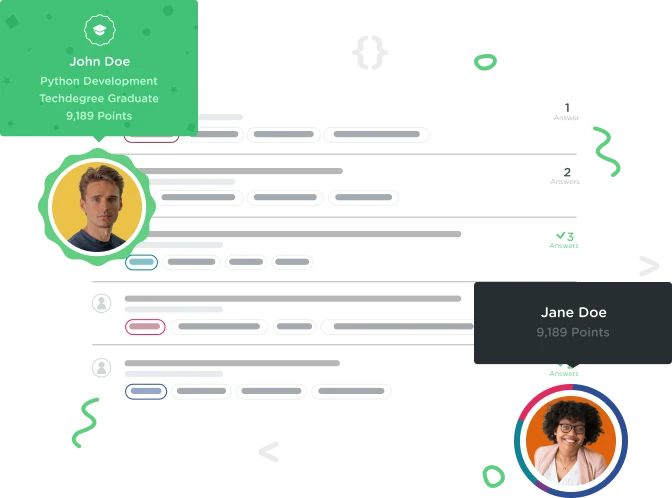

dima Dima
901 PointsWhat can I fix to get in working, any help would help
Create a function named word_count() that takes a string. Return a dictionary with each word in the string as the key and the number of times it appears as the value.
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(str1):
str2=str1.lower()
str3=list[str2.split]
count=0
for item in str3:
count=count+1
return new_dict{item:count}
3 Answers
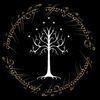
jacinator
11,936 PointsHi dima Dima,
This code should work for you. Comments explain some of it.
def word_count(str1):
str2 = str1.lower()
str3 = str2.split() # list[] doesn't work; list() around str.split() is redundant
dict1 = {} # Define dictionary to store information in
for word in str3:
try:
dict1[word] += 1 # Update...
except KeyError:
dict1[word] = 1 # ...or create information
return dict1 # Return stored information
# Alternate function is more concise
def word_count(string):
return_dict = dict()
for word in string.lower().split():
try:
return_dict[word] += 1
except KeyError:
return_dict[word] = 1
return return_dict

dima Dima
901 PointsThank you very much but can you help me understand, why do you write return_dict[word]=1, I understand the exception when the word realy appears only one time in the string, but what if, for example it appears three times. So for the first time and the second and third time the "try" part will work but then we should again break the loop to stop the counting but we cannot because the except part works only for the number one, and not the number three. So if this except part does not cover all the possible solutions, what does that? or how the except part does that?
Thanks in advance, Dima
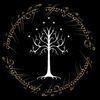
jacinator
11,936 Pointstry:
# This block will increase the word count if return_dict[word] already
# exists. Which means it has been counted once already.
return_dict[word] += 1
except KeyError:
# Otherwise this block will create the word as a key in the dictionary and
# assign it to being counted once.
return_dict[word] = 1
You shouldn't have to break the for
loop because it passes in each word in the string once. If it was a while
loop, you would have to break it eventually or it would continue indefinitely.
I hope that this helps explain a bit.

Benedictt Vasquez
2,130 PointsAquà otra manera.
def word_count(x):
y = ((x.lower()).split(' '))
dic = {}
for word in y:
dic.update((dict([(word, y.count(word))])))
return (dic)