Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial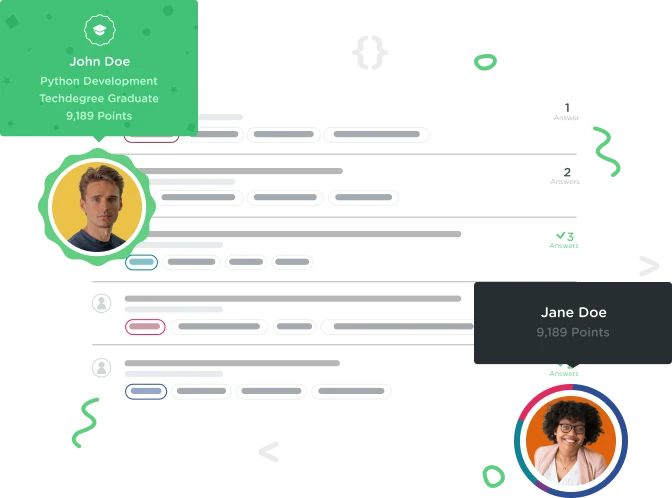
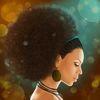
Angelic Thompson
2,017 PointsWhat did I do wrong?
Can someone explain because I think I got the gis of it
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public String getTileCount (){
String count"";
for (char tile: mHand.toCharArray())
{char display = 'R';
if (mHand.indexOf(tile) >= 0) {
display = tile;
}
count += display;
}
return count;
}
}
2 Answers
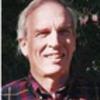
jcorum
71,830 PointsClose, but first, you need to take a tile as a parameter, rather than create it inside the method. Second, count should be an int, not a String, and the method needs to return an int. Third, when you do the for each loop, the variable t will become in turn each tile in the hand, so all you need do is compare it to the input parameter to see if they are equal, and if so, increment count.
public int getTileCount(char tile) {
int count = 0;
for (char t : mHand.toCharArray()) {
if (t == tile) {
count++;
}
}
return count;
}
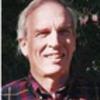
jcorum
71,830 PointsYes for your 1st item: Since the function needs to return a count, you need to create a variable to keep track of the count. And since it's a whole number it needs a type of Int.
Not quite for your 2nd item. For starters, there's no variable while. What you are counting are the number of tiles in the player's hand that equal the tile passed to the function as a parameter. So, for example, if the player's hand is X M V E I T G E, and the function is called this way: getTileCount('E'), you except the function to return the count of 2, as E occurs twice in the player's hand.
What the function does is look at each tile in the hand. The first time through the for loop the tile is X, and the if statement asks if X is equal to E (the tile passed in as a parameter). If it is, it increments count. If not it doesn't. Then, the second time through the loop it checks if M is equal to E, etc.
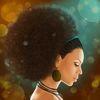
Angelic Thompson
2,017 Pointsokay thank you
Angelic Thompson
2,017 PointsAngelic Thompson
2,017 PointsSo: