Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial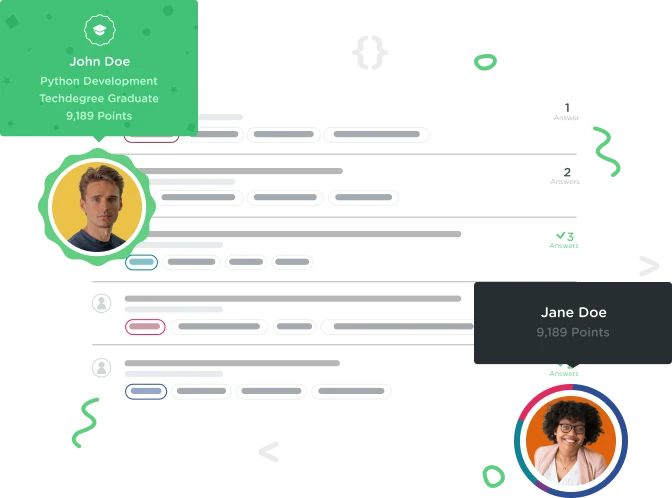

Ismael Soumahoro
6,410 PointsWhat did I do wrong
In this challenge, we have the following geographical coordinates
Eiffel Tower - lat: 48.8582, lon: 2.2945 Great Pyramid - lat: 29.9792, lon: 31.1344 Sydney Opera House - lat: 33.8587, lon: 151.2140
Declare a function named coordinates that takes a single parameter of type String, with an external name for, a local name of location, and returns a tuple containing two Double values (Note: You do not have to name the return values). For example, if I use your function and pass in the string "Eiffel Tower" as an argument, I should get (48.8582, 2.2945) as the value. If a string is passed in that doesnβt match the set above, return (0,0)
// Enter your code below
func coordinates(ofLocation location: String) -> (lat: Double, long: Double) {
var lat = 0.0
var long = 0.0
switch location {
case "Eiffel Tower": return(48.8582, 2.2945)
case "Great Pyramid": return(29.9792, 31.1344)
case "Sydney Opera House": return(33.8587, 151.2140)
default: return(0,0)
}
return(lat, long)
}
2 Answers
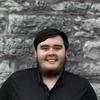
Michael Hulet
47,912 PointsThe challenge asks you to write a method with a signature of coordinates(for:)
, but yours is currently coordinates(ofLocation:)
. In Swift, parameters have names, but their name can be different both inside and outside the function. The external name is the first word in the parameter's declaration, and its internal name is the second. For example, if I wanted a function called exclamation
that took 1 parameter that I wanted to be called of
outside the function but phrase
inside the function, it'd look something like this:
func exclamation(of phrase: String) -> String{
return phrase + "!"
}
The above can be called like this:
exclamation(of: "I wrote a new program today")
//Returns "I wrote a new program today!"
Furthermore, this challenge says that "you do not have to name the return values", but what is mean is "please do not name the return values". I know what it says makes it sound like it'll take either named or unnamed values, but Treehouse challenges can be a bit finnicky like that sometimes, so it's best to follow their directions exactly, even if they say you don't have to. Once you fix the above and remove the names from the tuple that's return
ed, I think you'll be all set
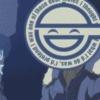
Jonathan Ruiz
2,998 PointsHi Ismael your close there is a few things in your code that don't need to be included though. In the parameters of the function you need to have the external name for and internal name location. Also you don't need to give names for the return values just make sure they return a tuple with two double values. It should look like this
fun coordinates (for location: String) -> (Double, Double) {
}
In the function you don't need to include the variable for lat and long. At the end when you type return (lat, long) its not necessary because while your function does need to return a tuple with two double values to run you are doing this in your switch statement. You wrote your switch statement perfectly. The code will end up looking like this.
func coordinates(for location: String) -> (Double, Double) {
switch location {
case "Eiffel Tower": return(48.8582, 2.2945)
case "Great Pyramid": return(29.9792, 31.1344)
case "Sydney Opera House": return(33.8587, 151.2140)
default: return(0,0)
}
}
Hope this helps !