Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial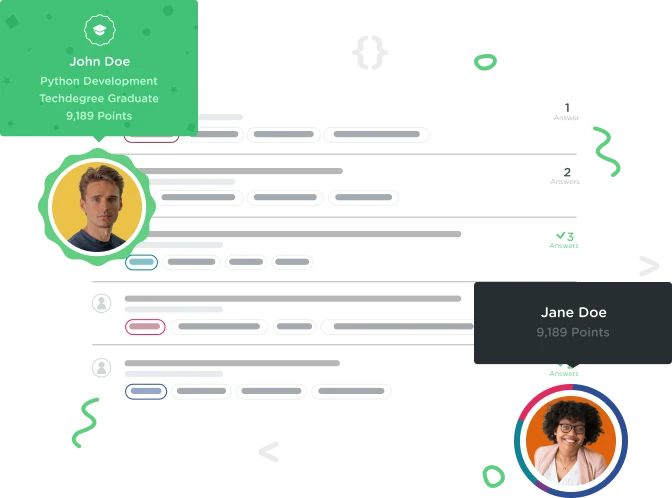

Gavril Florin Todoran
2,556 Pointswhat did I do wrong ?
I can't seem to understand the question
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive(int lapsToGo) {
lapsDriven += LapsDriven;
barCount -= barCount;
barCount == lapsToGo;
}
public void drive() {
lapsDriven++;
barCount--;
}
3 Answers
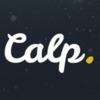
calp
10,317 PointsFor the first task, you need to change the drive method to accept the laps as a parameter and adjust the two variables accordingly.
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
}
For the second task you need to overload the drive method by adding another drive method that doesn't take a parameter. This method should call drive with a value of 1.
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
}
public void drive() {
drive(1);
}

Collin Halliday
Full Stack JavaScript Techdegree Student 17,491 PointsHey Gavril,
I agree that the question is a little confusing. It basically wants you, for the first task, to modify the original drive() method to take a parameter of int lapsToGo, and then to modify the lapsDriven and barCount variables accordingly in the method's body. In this case, you want to add the lapsToGo parameter to the lapsDriven variable, and subtract it from the barCount variable, using the appropriate += and -= operators.
Then, for the second task, the challenge is trying to get you to overload your new drive() method with another drive() method. Essentially, you need to create another drive() method without any parameters, and this new drive() method will simply call the other drive() method within its body, passing an int of 1 as its argument.
I hope that helps. Best of luck!

Gavril Florin Todoran
2,556 Pointsclass GoKart { public static final int MAX_BARS = 8; private String color; private int barCount; private int lapsDriven;
public GoKart(String color) { this.color = color; }
public String getColor() { return color; }
public void charge() { barCount = MAX_BARS; }
public boolean isBatteryEmpty() { return barCount == 0; }
public boolean isFullyCharged() { return barCount == MAX_BARS; }
public void drive(int laps) { lapsDriven += laps; barCount -= laps; }
public void drive() { drive(1); }
Same code if passed on Mac shows me "oops! it looks like task1 is no longer passing" while if the exact code is run on windows based machine passes with congrats, I can't understand this

Gavril Florin Todoran
2,556 PointsEven thou the syntax is correct on MAC this would not run for no reason while on the windows machine it does. Thank you all for your help. Much appreciated