Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial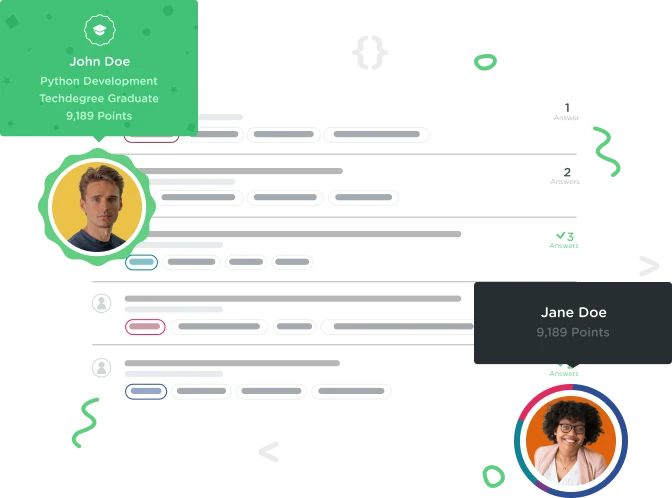

devante wallace
5,151 Pointswhat do i need to add to my code in order to make laps increase every time run_lap is run?
Im guessing an if statement or while loop but i dont know how to add it in?
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.length = length
self.fuel_remaining = self.fuel_remaining - self.length*0.125
self.laps =+ 1
3 Answers
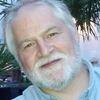
Jeff Muday
Treehouse Moderator 28,720 PointsYou're very close! The last instruction of the challenge is to set the class variable 'laps' inside of the the __init__
method.
A good programming practice is to set all instance variables (color, fuel_remaining, laps) inside of the __init__
. This prevents the parent class from changing the data inside the instance variables.
Good luck with your Python journey!
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
# set laps to 0 inside the __init__
self.laps = 0
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.length = length
self.fuel_remaining = self.fuel_remaining - self.length*0.125
self.laps += 1
[MOD: added backticks around `__init__
`-cf]

KRIS NIKOLAISEN
54,971 PointsThis
self.laps =+ 1
should be:
self.laps += 1

kevin curtis
15,287 PointsHi Devante, That challenge seems a bit buggy. I did the same challenge earlier today. It told me to try again even though the answer was correct. I rewrote the same code, clicked submit again and it went through ok for me.
if you've made the adjustments as Jeff suggested, then your code should be good to go.

devante wallace
5,151 Pointsok thank you
devante wallace
5,151 Pointsdevante wallace
5,151 PointsGreat thank you !
devante wallace
5,151 Pointsdevante wallace
5,151 PointsSo i have done this and it still says my code is incorrect, is there something else i am missing?