Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial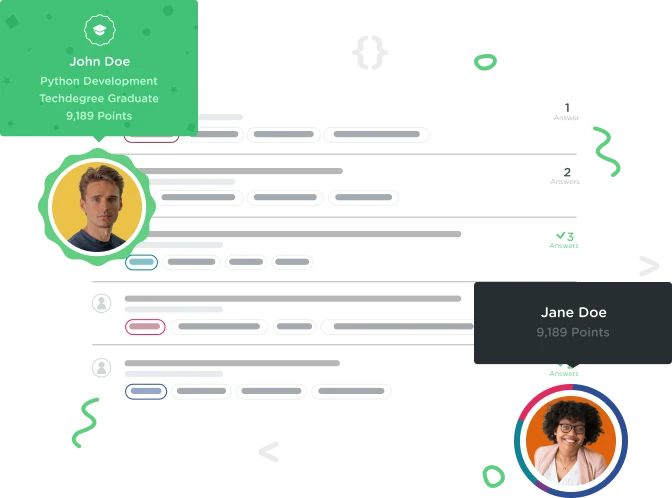
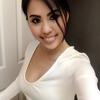
Vy Nguyen
4,350 PointsWhat do they mean by anonymous function?
Please help
//Problem: It look gross in smaller browser widths and small devices
//Solution: To hide the text links and swap them out with a more appropriate navigation
//Create a select and append to #menu
var $select = $("<select></select>");
$("#menu").append($select);
//Cycle over menu links
$("#menu a").each(function) {};
//Create an option
//option's value is the href
//option's text is the text of link
//append option to select
//Create button
//Bind click to button
//Go to select's location
//Modify CSS to hide links on small width and show button and select
//Also hides select and button on larger width and show's links
//Deal with selected options depending on current page
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<div id="menu">
<ul>
<li class="selected"><a href="index.html">Home</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
<li><a href="support.html">Support</a></li>
<li><a href="faqs.html">FAQs</a></li>
<li><a href="events.html">Events</a></li>
</ul>
</div>
<h1>Home</h1>
<p>This is the home page.</p>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
2 Answers

Brandon Benefield
7,739 PointsSo an anonymous function in JS is a function without a name and is stored in a variable.
Normal function
function hello() {
console.log('Hello');
}
hello(); //returns Hello
Anonymous function
const hello = function () {
console.log('Hello');
}
hello(); //returns Hello
Anonymous functions have the advantage of being passed along your code this way and making your code more succinct. Along with cleaner code, it also allows you to DRY your code.
If you are still a little fuzzy on the real difference go ahead and check out https://www.w3schools.com/js/js_function_definition.asp

justdave
31,518 PointsThey are essentially the same. By naming your function you make it available for reuse in the scope of where it was defined and have a way to call it directly.
You might have a bunch of functions defined within the scope. You can think about it as once they are defined there are just a bunch of functions hanging out waiting for you to put them to work. We give them names so that when you need to call them, they know which one you want. Essentially yelling at our group of functions "Hey, function xyz, time to come do your thing".
We don't need to give them a name if we are never going to call them directly, i.e they are part of another function. Then it's more like "Hey you, do your thing".